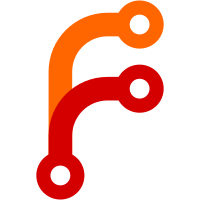
The repo path is typically relative, not absolute, so providing it to absPathFrom doesn't yield an absolute path. This is not a bug, just unclear documentation. Indeed, there seem to be no reason to simplifyPath here, which absPathFrom does, so instead just combine the repo path and the TopFilePath. Also, removed an export of the TopFilePath constructor; asTopFilePath is provided to construct one as-is.
77 lines
2.1 KiB
Haskell
77 lines
2.1 KiB
Haskell
{- git FilePath library
|
|
-
|
|
- Different git commands use different types of FilePaths to refer to
|
|
- files in the repository. Some commands use paths relative to the
|
|
- top of the repository even when run in a subdirectory. Adding some
|
|
- types helps keep that straight.
|
|
-
|
|
- Copyright 2012-2013 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module Git.FilePath (
|
|
TopFilePath,
|
|
getTopFilePath,
|
|
fromTopFilePath,
|
|
toTopFilePath,
|
|
asTopFilePath,
|
|
InternalGitPath,
|
|
toInternalGitPath,
|
|
fromInternalGitPath,
|
|
absoluteGitPath
|
|
) where
|
|
|
|
import Common
|
|
import Git
|
|
|
|
import qualified System.FilePath.Posix
|
|
|
|
{- A FilePath, relative to the top of the git repository. -}
|
|
newtype TopFilePath = TopFilePath { getTopFilePath :: FilePath }
|
|
deriving (Show)
|
|
|
|
{- Path to a TopFilePath, within the provided git repo. -}
|
|
fromTopFilePath :: TopFilePath -> Git.Repo -> FilePath
|
|
fromTopFilePath p repo = combine (repoPath repo) (getTopFilePath p)
|
|
|
|
{- The input FilePath can be absolute, or relative to the CWD. -}
|
|
toTopFilePath :: FilePath -> Git.Repo -> IO TopFilePath
|
|
toTopFilePath file repo = TopFilePath <$> relPathDirToFile (repoPath repo) file
|
|
|
|
{- The input FilePath must already be relative to the top of the git
|
|
- repository -}
|
|
asTopFilePath :: FilePath -> TopFilePath
|
|
asTopFilePath file = TopFilePath file
|
|
|
|
{- Git may use a different representation of a path when storing
|
|
- it internally.
|
|
-
|
|
- On Windows, git uses '/' to separate paths stored in the repository,
|
|
- despite Windows using '\'.
|
|
-
|
|
-}
|
|
type InternalGitPath = String
|
|
|
|
toInternalGitPath :: FilePath -> InternalGitPath
|
|
#ifndef mingw32_HOST_OS
|
|
toInternalGitPath = id
|
|
#else
|
|
toInternalGitPath = replace "\\" "/"
|
|
#endif
|
|
|
|
fromInternalGitPath :: InternalGitPath -> FilePath
|
|
#ifndef mingw32_HOST_OS
|
|
fromInternalGitPath = id
|
|
#else
|
|
fromInternalGitPath = replace "/" "\\"
|
|
#endif
|
|
|
|
{- isAbsolute on Windows does not think "/foo" or "\foo" is absolute,
|
|
- so try posix paths.
|
|
-}
|
|
absoluteGitPath :: FilePath -> Bool
|
|
absoluteGitPath p = isAbsolute p ||
|
|
System.FilePath.Posix.isAbsolute (toInternalGitPath p)
|