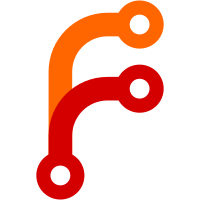
Rather than limiting it to PerformStage and CleanupStage, this opens it up so any number of stages can be added as needed by commands. Each concurrent command has a set of stages that it uses, and only transitions between those can block waiting for a free slot in the worker pool. Calling enteringStage for some other stage does not block, and has very little overhead. Note that while before the Annex state was duplicated on the first call to commandAction, this now happens earlier, in startConcurrency. That means that seek stage actions should that use startConcurrency and then modify Annex state won't modify the state of worker threads they then start. I audited all of them, and only Command.Seek did so; prepMerge changes the working directory and so has to come before startConcurrency. Also, the remote list is built before duplicating the state, which means that it gets built earlier now than it used to. This would only have an effect of making commands that end up not needing to perform any actions unncessary build the remote list (only when they're run with concurrency enable), but that's a minor overhead compared to commands seeking through the work tree and determining they don't need to do anything.
74 lines
2.2 KiB
Haskell
74 lines
2.2 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Copy where
|
|
|
|
import Command
|
|
import qualified Command.Move
|
|
import qualified Remote
|
|
import Annex.Wanted
|
|
import Annex.NumCopies
|
|
|
|
cmd :: Command
|
|
cmd = withGlobalOptions [jobsOption, jsonOptions, jsonProgressOption, annexedMatchingOptions] $
|
|
command "copy" SectionCommon
|
|
"copy content of files to/from another repository"
|
|
paramPaths (seek <--< optParser)
|
|
|
|
data CopyOptions = CopyOptions
|
|
{ copyFiles :: CmdParams
|
|
, fromToOptions :: FromToHereOptions
|
|
, keyOptions :: Maybe KeyOptions
|
|
, autoMode :: Bool
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser CopyOptions
|
|
optParser desc = CopyOptions
|
|
<$> cmdParams desc
|
|
<*> parseFromToHereOptions
|
|
<*> optional (parseKeyOptions <|> parseFailedTransfersOption)
|
|
<*> parseAutoOption
|
|
<*> parseBatchOption
|
|
|
|
instance DeferredParseClass CopyOptions where
|
|
finishParse v = CopyOptions
|
|
<$> pure (copyFiles v)
|
|
<*> finishParse (fromToOptions v)
|
|
<*> pure (keyOptions v)
|
|
<*> pure (autoMode v)
|
|
<*> pure (batchOption v)
|
|
|
|
seek :: CopyOptions -> CommandSeek
|
|
seek o = startConcurrency commandStages $ do
|
|
let go = whenAnnexed $ start o
|
|
case batchOption o of
|
|
Batch fmt -> batchFilesMatching fmt go
|
|
NoBatch -> withKeyOptions
|
|
(keyOptions o) (autoMode o)
|
|
(commandAction . Command.Move.startKey (fromToOptions o) Command.Move.RemoveNever)
|
|
(withFilesInGit $ commandAction . go)
|
|
=<< workTreeItems (copyFiles o)
|
|
|
|
{- A copy is just a move that does not delete the source file.
|
|
- However, auto mode avoids unnecessary copies, and avoids getting or
|
|
- sending non-preferred content. -}
|
|
start :: CopyOptions -> FilePath -> Key -> CommandStart
|
|
start o file key = stopUnless shouldCopy $
|
|
Command.Move.start (fromToOptions o) Command.Move.RemoveNever file key
|
|
where
|
|
shouldCopy
|
|
| autoMode o = want <||> numCopiesCheck file key (<)
|
|
| otherwise = return True
|
|
want = case fromToOptions o of
|
|
Right (ToRemote dest) ->
|
|
(Remote.uuid <$> getParsed dest) >>= checkwantsend
|
|
Right (FromRemote _) -> checkwantget
|
|
Left ToHere -> checkwantget
|
|
|
|
checkwantsend = wantSend False (Just key) (AssociatedFile (Just file))
|
|
checkwantget = wantGet False (Just key) (AssociatedFile (Just file))
|