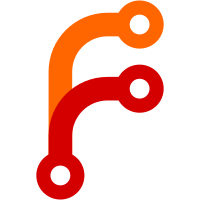
This patch avoids main process never response back to renderer if the options is invalid.
2.4 KiB
2.4 KiB
desktop-capturer
The desktop-capturer
is a renderer module used to capture the content of
screen and individual app windows.
// In the renderer process.
var desktopCapturer = require('desktop-capturer');
desktopCapturer.getSources({types: ['window', 'screen']}, function(error, sources) {
if (error) throw error;
for (var i = 0; i < sources.length; ++i) {
if (sources[i].name == "Electron") {
navigator.webkitGetUserMedia({
audio: false,
video: {
mandatory: {
chromeMediaSource: 'desktop',
chromeMediaSourceId: sources[i].id,
minWidth: 1280,
maxWidth: 1280,
minHeight: 720,
maxHeight: 720
}
}
}, gotStream, getUserMediaError);
return;
}
}
});
function gotStream(stream) {
document.querySelector('video').src = URL.createObjectURL(stream);
}
function getUserMediaError(e) {
console.log('getUserMediaError');
}
Methods
The desktopCapturer
module has the following methods:
desktopCapturer.getSources(options, callback)
options
Object, properties:
types
Array - An array of String that enums the types of desktop sources.screen
String - Screenwindow
String - Individual window
thumbnailSize
Object (optional) - The suggested size that thumbnail should be scaled.width
Integer - The width of thumbnail. By default, it is 150px.height
Integer - The height of thumbnail. By default, it is 150px.
callback
Function - function(error, sources) {}
error
Errorsources
Array - An array of Source
Gets all desktop sources.
Note: There is no garuantee that the size of source.thumbnail
is always
the same as the thumnbailSize
in options
. It also depends on the scale of
the screen or window.
Source
Source
is an object represents a captured screen or individual window. It has
following properties:
id
String - The id of the captured window or screen used innavigator.webkitGetUserMedia
. The format looks like 'window:XX' or 'screen:XX' where XX is a random generated number.name
String - The descriped name of the capturing screen or window. If the source is a screen, the name will be 'Entire Screen' or 'Screen '; if it is a window, the name will be the window's title.thumbnail
NativeImage - A thumbnail image.