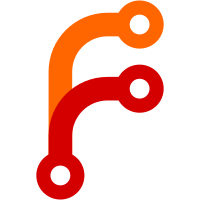
* docs: add references to app.whenReady() in isReady * refactor: prefer app.whenReady() In the docs, specs, and lib, replace instances of `app.once('ready')` (seen occasionally) and `app.on('ready')` (extremely common) with `app.whenReady()`. It's better to encourage users to use whenReady(): 1. it handles the edge case of registering for 'ready' after it's fired 2. it avoids the minor wart of leaving an active listener alive for an event that wll never fire again
55 lines
1.8 KiB
Markdown
55 lines
1.8 KiB
Markdown
# netLog
|
|
|
|
> Logging network events for a session.
|
|
|
|
Process: [Main](../glossary.md#main-process)
|
|
|
|
```javascript
|
|
const { netLog } = require('electron')
|
|
|
|
app.whenReady().then(async () => {
|
|
await netLog.startLogging('/path/to/net-log')
|
|
// After some network events
|
|
const path = await netLog.stopLogging()
|
|
console.log('Net-logs written to', path)
|
|
})
|
|
```
|
|
|
|
See [`--log-net-log`](command-line-switches.md#--log-net-logpath) to log network events throughout the app's lifecycle.
|
|
|
|
**Note:** All methods unless specified can only be used after the `ready` event
|
|
of the `app` module gets emitted.
|
|
|
|
## Methods
|
|
|
|
### `netLog.startLogging(path[, options])`
|
|
|
|
* `path` String - File path to record network logs.
|
|
* `options` Object (optional)
|
|
* `captureMode` String (optional) - What kinds of data should be captured. By
|
|
default, only metadata about requests will be captured. Setting this to
|
|
`includeSensitive` will include cookies and authentication data. Setting
|
|
it to `everything` will include all bytes transferred on sockets. Can be
|
|
`default`, `includeSensitive` or `everything`.
|
|
* `maxFileSize` Number (optional) - When the log grows beyond this size,
|
|
logging will automatically stop. Defaults to unlimited.
|
|
|
|
Returns `Promise<void>` - resolves when the net log has begun recording.
|
|
|
|
Starts recording network events to `path`.
|
|
|
|
### `netLog.stopLogging()`
|
|
|
|
Returns `Promise<String>` - resolves with a file path to which network logs were recorded.
|
|
|
|
Stops recording network events. If not called, net logging will automatically end when app quits.
|
|
|
|
## Properties
|
|
|
|
### `netLog.currentlyLogging` _Readonly_
|
|
|
|
A `Boolean` property that indicates whether network logs are recorded.
|
|
|
|
### `netLog.currentlyLoggingPath` _Readonly_ _Deprecated_
|
|
|
|
A `String` property that returns the path to the current log file.
|