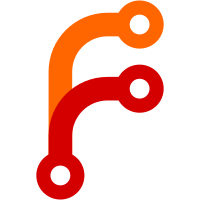
* fix: ensure the typescript definitions only export correct value types In typescript there are two main types of "types" you can export, value types (class, const) and definition types (type, interface). The typescript compiler will let anything declared via const or class be used as a value. Unfortunately we were exporting a bunch of things (see the diff) as class/const when they weren't actually exported values. This lead to typescript being happy but the runtime throwing errors (not something we want). This change passes "exported-in" context through our docs, to the parser and then to the definitions generator to ensure we only mark things as exported in the ts defs that we actually export. Fixes #22167 * chore: update typescript-defs * chore: update typescript-defs * chore: fix bad typescript in IPC test * docs: test rendering of new syntax * chore: update per feedback, use same syntax but with 'this is not exportedd' line
73 lines
2.8 KiB
Markdown
73 lines
2.8 KiB
Markdown
## Class: ServiceWorkers
|
|
|
|
> Query and receive events from a sessions active service workers.
|
|
|
|
Process: [Main](../glossary.md#main-process)<br />
|
|
_This class is not exported from the `'electron'` module. It is only available as a return value of other methods in the Electron API._
|
|
|
|
Instances of the `ServiceWorkers` class are accessed by using `serviceWorkers` property of
|
|
a `Session`.
|
|
|
|
For example:
|
|
|
|
```javascript
|
|
const { session } = require('electron')
|
|
|
|
// Get all service workers.
|
|
console.log(session.defaultSession.serviceWorkers.getAllRunning())
|
|
|
|
// Handle logs and get service worker info
|
|
session.defaultSession.serviceWorkers.on('console-message', (event, messageDetails) => {
|
|
console.log(
|
|
'Got service worker message',
|
|
messageDetails,
|
|
'from',
|
|
session.defaultSession.serviceWorkers.getFromVersionID(messageDetails.versionId)
|
|
)
|
|
})
|
|
```
|
|
|
|
### Instance Events
|
|
|
|
The following events are available on instances of `ServiceWorkers`:
|
|
|
|
#### Event: 'console-message'
|
|
|
|
Returns:
|
|
|
|
* `event` Event
|
|
* `messageDetails` Object - Information about the console message
|
|
* `message` String - The actual console message
|
|
* `versionId` Number - The version ID of the service worker that sent the log message
|
|
* `source` String - The type of source for this message. Can be `javascript`, `xml`, `network`, `console-api`, `storage`, `app-cache`, `rendering`, `security`, `deprecation`, `worker`, `violation`, `intervention`, `recommendation` or `other`.
|
|
* `level` Number - The log level, from 0 to 3. In order it matches `verbose`, `info`, `warning` and `error`.
|
|
* `sourceUrl` String - The URL the message came from
|
|
* `lineNumber` Number - The line number of the source that triggered this console message
|
|
|
|
Emitted when a service worker logs something to the console.
|
|
|
|
#### Event: 'registration-completed'
|
|
|
|
Returns:
|
|
|
|
* `event` Event
|
|
* `details` Object - Information about the registered service worker
|
|
* `scope` String - The base URL that a service worker is registered for
|
|
|
|
Emitted when a service worker has been registered. Can occur after a call to [`navigator.serviceWorker.register('/sw.js')`](https://developer.mozilla.org/en-US/docs/Web/API/ServiceWorkerContainer/register) successfully resolves or when a Chrome extension is loaded.
|
|
|
|
### Instance Methods
|
|
|
|
The following methods are available on instances of `ServiceWorkers`:
|
|
|
|
#### `serviceWorkers.getAllRunning()`
|
|
|
|
Returns `Record<Number, ServiceWorkerInfo>` - A [ServiceWorkerInfo](structures/service-worker-info.md) object where the keys are the service worker version ID and the values are the information about that service worker.
|
|
|
|
#### `serviceWorkers.getFromVersionID(versionId)`
|
|
|
|
* `versionId` Number
|
|
|
|
Returns [`ServiceWorkerInfo`](structures/service-worker-info.md) - Information about this service worker
|
|
|
|
If the service worker does not exist or is not running this method will throw an exception.
|