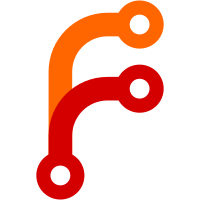
- New flat theme (with padding tightened a bit from the default to fit in right-hand pane) - Adds search/replace within notes - Adds URL autolinking - Image pasting/dragging is now properly disallowed (though TinyMCE 4 has hooks that may allow us to actually support this by automatically creating attachments) - New blockquote style with color bar - Replaces custom context menu on link click with built-in version To-do: - Fix display of pop-ups, which are now modal dialogs within the note frame instead of pop-up windows, to stay fully within the frame - Localize (more important now that there are tooltips) - Support image dragging - Update elements list for HTML5, for better drag-and-drop? - Move directionality control to context menu instead of taking up toolbar space? - Evaluate other plugins for potential inclusion - Show additional controls in separate note window? - Fix opacity of text in tooltips Closes #451, closes #421
116 lines
No EOL
2.6 KiB
JavaScript
116 lines
No EOL
2.6 KiB
JavaScript
/**
|
|
* plugin.js
|
|
*
|
|
* Released under LGPL License.
|
|
* Copyright (c) 1999-2015 Ephox Corp. All rights reserved
|
|
*
|
|
* License: http://www.tinymce.com/license
|
|
* Contributing: http://www.tinymce.com/contributing
|
|
*/
|
|
|
|
/*global tinymce:true */
|
|
|
|
tinymce.PluginManager.add('contextmenu', function(editor) {
|
|
var menu, visibleState, contextmenuNeverUseNative = editor.settings.contextmenu_never_use_native;
|
|
|
|
var isNativeOverrideKeyEvent = function (e) {
|
|
return e.ctrlKey && !contextmenuNeverUseNative;
|
|
};
|
|
|
|
var isMacWebKit = function () {
|
|
return tinymce.Env.mac && tinymce.Env.webkit;
|
|
};
|
|
|
|
var isContextMenuVisible = function () {
|
|
return visibleState === true;
|
|
};
|
|
|
|
/**
|
|
* This takes care of a os x native issue where it expands the selection
|
|
* to the word at the caret position to do "lookups". Since we are overriding
|
|
* the context menu we also need to override this expanding so the behavior becomes
|
|
* normalized. Firefox on os x doesn't expand to the word when using the context menu.
|
|
*/
|
|
editor.on('mousedown', function (e) {
|
|
if (isMacWebKit() && e.button === 2 && !isNativeOverrideKeyEvent(e)) {
|
|
if (editor.selection.isCollapsed()) {
|
|
editor.once('contextmenu', function (e) {
|
|
editor.selection.placeCaretAt(e.clientX, e.clientY);
|
|
});
|
|
}
|
|
}
|
|
});
|
|
|
|
editor.on('contextmenu', function(e) {
|
|
var contextmenu;
|
|
|
|
if (isNativeOverrideKeyEvent(e)) {
|
|
return;
|
|
}
|
|
|
|
e.preventDefault();
|
|
contextmenu = editor.settings.contextmenu || 'link openlink image inserttable | cell row column deletetable';
|
|
|
|
// Render menu
|
|
if (!menu) {
|
|
var items = [];
|
|
|
|
tinymce.each(contextmenu.split(/[ ,]/), function(name) {
|
|
var item = editor.menuItems[name];
|
|
|
|
if (name == '|') {
|
|
item = {text: name};
|
|
}
|
|
|
|
if (item) {
|
|
item.shortcut = ''; // Hide shortcuts
|
|
items.push(item);
|
|
}
|
|
});
|
|
|
|
for (var i = 0; i < items.length; i++) {
|
|
if (items[i].text == '|') {
|
|
if (i === 0 || i == items.length - 1) {
|
|
items.splice(i, 1);
|
|
}
|
|
}
|
|
}
|
|
|
|
menu = new tinymce.ui.Menu({
|
|
items: items,
|
|
context: 'contextmenu',
|
|
classes: 'contextmenu'
|
|
}).renderTo();
|
|
|
|
menu.on('hide', function (e) {
|
|
if (e.control === this) {
|
|
visibleState = false;
|
|
}
|
|
});
|
|
|
|
editor.on('remove', function() {
|
|
menu.remove();
|
|
menu = null;
|
|
});
|
|
|
|
} else {
|
|
menu.show();
|
|
}
|
|
|
|
// Position menu
|
|
var pos = {x: e.pageX, y: e.pageY};
|
|
|
|
if (!editor.inline) {
|
|
pos = tinymce.DOM.getPos(editor.getContentAreaContainer());
|
|
pos.x += e.clientX;
|
|
pos.y += e.clientY;
|
|
}
|
|
|
|
menu.moveTo(pos.x, pos.y);
|
|
visibleState = true;
|
|
});
|
|
|
|
return {
|
|
isContextMenuVisible: isContextMenuVisible
|
|
};
|
|
}); |