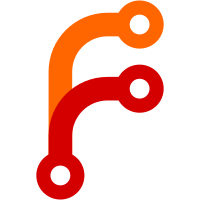
- Currently enabled only for ScienceDirect. Can be enabled via a whitelist - Matches the HiddenBrowser loaded HTML page for a captcha element. If the captcha element class changes, this will break (but the alternative is potentially displaying a captcha clearing window when something else that is not a captcha guard is loaded). - Captcha clear timeout for 60s. - Doesn't automatically switch focus back to the browser which intiated the item save via the Connector. - Stores the cookies used to clear the captcha for future saves from the same domain. Discards Connector supplied User Agent, since CF bot detector checks UA header against actual UA behavior like TLS handshake and if the UA acts different to what it's supposed to, the bot challenge is not cleared. Other changes: - Adjusted the cookie sandbox to allow multiple cookie sandboxes to be active (and simplified some legacy code that was meant to cover a bug in old FX codebase). - HiddenBrowser API changed to be Object oriented, translator tester in the translate repo will need to be updated after a merge (have the change ready). - Improved Connector Server attachment progress handling
60 lines
2.4 KiB
JavaScript
60 lines
2.4 KiB
JavaScript
/*
|
|
***** BEGIN LICENSE BLOCK *****
|
|
|
|
Copyright © 2023 Corporation for Digital Scholarship
|
|
Vienna, Virginia, USA
|
|
http://zotero.org
|
|
|
|
This file is part of Zotero.
|
|
|
|
Zotero is free software: you can redistribute it and/or modify
|
|
it under the terms of the GNU Affero General Public License as published by
|
|
the Free Software Foundation, either version 3 of the License, or
|
|
(at your option) any later version.
|
|
|
|
Zotero is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU Affero General Public License for more details.
|
|
|
|
You should have received a copy of the GNU Affero General Public License
|
|
along with Zotero. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
***** END LICENSE BLOCK *****
|
|
*/
|
|
|
|
describe("Zotero.BrowserDownload", function () {
|
|
describe("#downloadPDF()", function () {
|
|
var tmpFile = Zotero.getTempDirectory();
|
|
tmpFile.append('browserDownloadTest.pdf');
|
|
|
|
it("#downloadPDF() should download a PDF from a JS redirect page", async function () {
|
|
this.timeout(65e3);
|
|
|
|
await Zotero.BrowserDownload.downloadPDF('https://zotero-static.s3.amazonaws.com/test-pdf-redirect.html', tmpFile.path);
|
|
|
|
var sample = await Zotero.File.getContentsAsync(tmpFile, null, 1000);
|
|
assert.equal(Zotero.MIME.sniffForMIMEType(sample), 'application/pdf');
|
|
});
|
|
|
|
// Needs a js-redirect delay in test-pdf-redirect.html
|
|
it.skip("should display a viewer to clear a captcha if detected", async function () {
|
|
// Make it so that downloadPDF() times out with a hidden browser, which simulates running into a captcha
|
|
Zotero.Prefs.set('downloadPDFViaBrowser.downloadTimeout', 10);
|
|
let downloadPDFStub = sinon.stub(Zotero.BrowserDownload, "downloadPDFViaViewer");
|
|
|
|
let promise = Zotero.BrowserDownload.downloadPDF('https://zotero-static.s3.amazonaws.com/test-pdf-redirect.html', tmpFile.path,
|
|
{ cookieSandbox: new Zotero.CookieSandbox(), shouldDisplayCaptcha: true });
|
|
await new Promise(resolve => downloadPDFStub.callsFake((...args) => {
|
|
resolve();
|
|
Zotero.Prefs.set('downloadPDFViaBrowser.downloadTimeout', 60e3);
|
|
return downloadPDFStub.wrappedMethod.call(Zotero.BrowserDownload, ...args);
|
|
}));
|
|
|
|
await promise;
|
|
|
|
assert.isTrue(downloadPDFStub.calledOnce);
|
|
downloadPDFStub.restore();
|
|
});
|
|
});
|
|
});
|