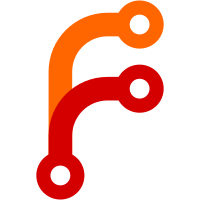
Previously, objects were first downloaded and saved to the sync cache, which was then processed separately to create/update local objects. This meant that a server bug could result in invalid data in the sync cache that would never be processed. Now, objects are saved as they're downloaded and only added to the sync cache after being successfully saved. The keys of objects that fail are added to a queue, and those objects are refetched and retried on a backoff schedule or when a new client version is installed (in case of a client bug or a client with outdated data model support). An alternative would be to save to the sync cache first and evict objects that fail and add them to the queue, but that requires more complicated logic, and it probably makes more sense just to buffer a few downloads ahead so that processing is never waiting for downloads to finish.
48 lines
1.6 KiB
JavaScript
48 lines
1.6 KiB
JavaScript
describe("Zotero.Schema", function() {
|
|
describe("#initializeSchema()", function () {
|
|
it("should set last client version", function* () {
|
|
yield resetDB({
|
|
thisArg: this,
|
|
skipBundledFiles: true
|
|
});
|
|
|
|
var sql = "SELECT value FROM settings WHERE setting='client' AND key='lastVersion'";
|
|
var lastVersion = yield Zotero.DB.valueQueryAsync(sql);
|
|
yield assert.eventually.equal(Zotero.DB.valueQueryAsync(sql), Zotero.version);
|
|
});
|
|
});
|
|
|
|
describe("#updateSchema()", function () {
|
|
it("should set last client version", function* () {
|
|
var sql = "REPLACE INTO settings (setting, key, value) VALUES ('client', 'lastVersion', ?)";
|
|
return Zotero.DB.queryAsync(sql, "5.0old");
|
|
|
|
yield Zotero.Schema.updateSchema();
|
|
|
|
var sql = "SELECT value FROM settings WHERE setting='client' AND key='lastVersion'";
|
|
var lastVersion = yield Zotero.DB.valueQueryAsync(sql);
|
|
yield assert.eventually.equal(Zotero.DB.valueQueryAsync(sql), Zotero.version);
|
|
});
|
|
});
|
|
|
|
describe("#integrityCheck()", function () {
|
|
before(function* () {
|
|
yield resetDB({
|
|
thisArg: this,
|
|
skipBundledFiles: true
|
|
});
|
|
})
|
|
|
|
it("should repair a foreign key violation", function* () {
|
|
yield assert.eventually.isTrue(Zotero.Schema.integrityCheck());
|
|
|
|
yield Zotero.DB.queryAsync("PRAGMA foreign_keys = OFF");
|
|
yield Zotero.DB.queryAsync("INSERT INTO itemTags VALUES (1234,1234,0)");
|
|
yield Zotero.DB.queryAsync("PRAGMA foreign_keys = ON");
|
|
|
|
yield assert.eventually.isFalse(Zotero.Schema.integrityCheck());
|
|
yield assert.eventually.isTrue(Zotero.Schema.integrityCheck(true));
|
|
yield assert.eventually.isTrue(Zotero.Schema.integrityCheck());
|
|
})
|
|
})
|
|
})
|