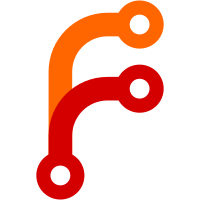
More details coming on Basecamp: http://chnm.grouphub.com/W161222 *** Important note: after checking out this code, be sure to delete the compreg.dat and xpti.dat files in your FF profile directory or else the extension will not load. They'll be regenerated when you start FF again, and you won't have to do it again unless we add interfaces or other components. Once I set up the XPI packaging system, this step won't be necessary. *** - Localization properties are now loaded directly via nsIStringBundleService and available via Scholar.getString(name) -- removed stringbundle from XUL - Updated status line in bottom right to reflect whether Scholar is loaded correctly or not (temporary, obviously)
121 lines
3.1 KiB
JavaScript
121 lines
3.1 KiB
JavaScript
const SCHOLAR_CONTRACTID = '@chnm.gmu.edu/Scholar;1';
|
|
const SCHOLAR_CLASSNAME = 'Firefox Scholar';
|
|
const SCHOLAR_CID = Components.ID('{e4c61080-ec2d-11da-8ad9-0800200c9a66}');
|
|
const SCHOLAR_IID = Components.interfaces.chnmIScholarService;
|
|
|
|
const Cc = Components.classes;
|
|
const Ci = Components.interfaces;
|
|
|
|
// Assign the global scope to a variable to passed via wrappedJSObject
|
|
var ScholarWrapped = this;
|
|
|
|
|
|
/********************************************************************
|
|
* Include the core objects to be stored within XPCOM
|
|
*********************************************************************/
|
|
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://scholar/content/overlay.js");
|
|
|
|
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://scholar/content/db.js");
|
|
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://scholar/content/data_access.js");
|
|
|
|
/********************************************************************/
|
|
|
|
|
|
// Initialize the Scholar service
|
|
//
|
|
// This runs when ScholarService is first requested.
|
|
// Calls to other XPCOM components must be in here rather than in top-level
|
|
// code, as other components may not have yet been initialized.
|
|
function setupService(){
|
|
Scholar.init();
|
|
}
|
|
|
|
function ScholarService(){
|
|
this.wrappedJSObject = ScholarWrapped.Scholar;
|
|
setupService();
|
|
}
|
|
|
|
|
|
/**
|
|
* Convenience method to replicate window.alert()
|
|
**/
|
|
function alert(msg){
|
|
Cc["@mozilla.org/embedcomp/prompt-service;1"]
|
|
.getService(Ci.nsIPromptService)
|
|
.alert(null, "", msg);
|
|
}
|
|
|
|
|
|
//
|
|
// XPCOM goop
|
|
//
|
|
ScholarService.prototype = {
|
|
QueryInterface: function(iid){
|
|
if (!iid.equals(Components.interfaces.nsISupports) &&
|
|
!iid.equals(SCHOLAR_IID)){
|
|
throw Components.results.NS_ERROR_NO_INTERFACE;
|
|
}
|
|
return this;
|
|
}
|
|
};
|
|
|
|
|
|
var ScholarFactory = {
|
|
createInstance: function(outer, iid){
|
|
if (outer != null){
|
|
throw Components.results.NS_ERROR_NO_AGGREGATION;
|
|
}
|
|
return new ScholarService().QueryInterface(iid);
|
|
}
|
|
};
|
|
|
|
|
|
var ScholarModule = {
|
|
_firstTime: true,
|
|
|
|
registerSelf: function(compMgr, fileSpec, location, type){
|
|
if (!this._firstTime){
|
|
throw Components.results.NS_ERROR_FACTORY_REGISTER_AGAIN;
|
|
}
|
|
this._firstTime = false;
|
|
|
|
compMgr =
|
|
compMgr.QueryInterface(Components.interfaces.nsIComponentRegistrar);
|
|
|
|
compMgr.registerFactoryLocation(SCHOLAR_CID,
|
|
SCHOLAR_CLASSNAME,
|
|
SCHOLAR_CONTRACTID,
|
|
fileSpec,
|
|
location,
|
|
type);
|
|
},
|
|
|
|
unregisterSelf: function(compMgr, location, type){
|
|
compMgr =
|
|
compMgr.QueryInterface(Components.interfaces.nsIComponentRegistrar);
|
|
compMgr.unregisterFactoryLocation(SCHOLAR_CID, location);
|
|
},
|
|
|
|
getClassObject: function(compMgr, cid, iid){
|
|
if (!cid.equals(SCHOLAR_CID)){
|
|
throw Components.results.NS_ERROR_NO_INTERFACE;
|
|
}
|
|
if (!iid.equals(Components.interfaces.nsIFactory)){
|
|
throw Components.results.NS_ERROR_NOT_IMPLEMENTED;
|
|
}
|
|
return ScholarFactory;
|
|
},
|
|
|
|
canUnload: function(compMgr){ return true; }
|
|
};
|
|
|
|
function NSGetModule(comMgr, fileSpec){ return ScholarModule; }
|