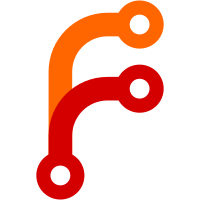
closes #100, migrate ingester to Scholar.Translate closes #88, migrate scrapers away from RDF closes #9, pull out LC subject heading tags references #87, add fromArray() and toArray() methods to item objects API changes: all translation (import/export/web) now goes through Scholar.Translate all Scholar-specific functions in scrapers start with "Scholar." rather than the jumbled up piggy bank un-namespaced confusion scrapers now longer specify items through RDF (the beginning of an item.fromArray()-like function exists in Scholar.Translate.prototype._itemDone()) scrapers can be any combination of import, export, and web (type is the sum of 1/2/4 respectively) scrapers now contain functions (doImport, doExport, doWeb) rather than loose code scrapers can call functions in other scrapers or just call the function to translate itself export accesses items item-by-item, rather than accepting a huge array of items MARC functions are now in the MARC import translator, and accessed by the web translators new features: import now works rudimentary RDF (unqualified dublin core only), RIS, and MARC import translators are implemented (although they are a little picky with respect to file extensions at the moment) items appear as they are scraped MARC import translator pulls out tags, although this seems to slow things down no icon appears next to a the URL when Scholar hasn't detected metadata, since this seemed somewhat confusing apologizes for the size of this diff. i figured if i was going to re-write the API, i might as well do it all at once and get everything working right.
64 lines
No EOL
2 KiB
JavaScript
64 lines
No EOL
2 KiB
JavaScript
Scholar_File_Interface = new function() {
|
|
this.exportFile = exportFile;
|
|
this.importFile = importFile;
|
|
|
|
/*
|
|
* Creates Scholar.Translate instance and shows file picker for file export
|
|
*/
|
|
function exportFile() {
|
|
var translation = new Scholar.Translate("export");
|
|
var translators = translation.getTranslators();
|
|
|
|
const nsIFilePicker = Components.interfaces.nsIFilePicker;
|
|
var fp = Components.classes["@mozilla.org/filepicker;1"]
|
|
.createInstance(nsIFilePicker);
|
|
fp.init(window, "Export", nsIFilePicker.modeSave);
|
|
for(var i in translators) {
|
|
fp.appendFilter(translators[i].label, translators[i].target);
|
|
}
|
|
var rv = fp.show();
|
|
if (rv == nsIFilePicker.returnOK || rv == nsIFilePicker.returnReplace) {
|
|
translation.setLocation(fp.file);
|
|
translation.setTranslator(translators[fp.filterIndex]);
|
|
//translation.setHandler("done", _exportDone);
|
|
translation.translate();
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Creates Scholar.Translate instance and shows file picker for file import
|
|
*/
|
|
function importFile() {
|
|
var translation = new Scholar.Translate("import");
|
|
var translators = translation.getTranslators();
|
|
|
|
const nsIFilePicker = Components.interfaces.nsIFilePicker;
|
|
var fp = Components.classes["@mozilla.org/filepicker;1"]
|
|
.createInstance(nsIFilePicker);
|
|
fp.init(window, "Import", nsIFilePicker.modeOpen);
|
|
for(var i in translators) {
|
|
fp.appendFilter(translators[i].label, "*."+translators[i].target);
|
|
}
|
|
|
|
var rv = fp.show();
|
|
if (rv == nsIFilePicker.returnOK || rv == nsIFilePicker.returnReplace) {
|
|
translation.setLocation(fp.file);
|
|
// get translators again, bc now we can check against the file
|
|
translators = translation.getTranslators();
|
|
if(translators.length) {
|
|
// TODO: display a list of available translators
|
|
translation.setTranslator(translators[0]);
|
|
translation.setHandler("itemDone", _importItemDone);
|
|
translation.translate();
|
|
}
|
|
}
|
|
}
|
|
|
|
/*
|
|
* Saves items after they've been imported. We could have a nice little
|
|
* "items imported" indicator, too.
|
|
*/
|
|
function _importItemDone(obj, item) {
|
|
item.save();
|
|
}
|
|
} |