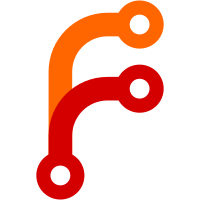
Fix a couple cases of lost text field focus after an edit, including focusing of the Title field after using New Item when a field is already being edited and has a changed value. Also, in tests, select My Library and wait for items to load when using the loadZoteroPane() support function. We could add a parameter to skip that or move it to a separate function, but the code to detect it is a bit convoluted and it's a prerequisite for many tests, so it's handy to have a function for it.
37 lines
1.2 KiB
JavaScript
37 lines
1.2 KiB
JavaScript
describe("ZoteroPane", function() {
|
|
var win, doc, zp;
|
|
|
|
// Load Zotero pane and select library
|
|
before(function* () {
|
|
win = yield loadZoteroPane();
|
|
doc = win.document;
|
|
zp = win.ZoteroPane;
|
|
});
|
|
|
|
after(function () {
|
|
win.close();
|
|
});
|
|
|
|
describe("#newItem", function () {
|
|
it("should create an item and focus the title field", function* () {
|
|
yield zp.newItem(Zotero.ItemTypes.getID('book'), {}, null, true);
|
|
var itemBox = doc.getElementById('zotero-editpane-item-box');
|
|
var textboxes = doc.getAnonymousNodes(itemBox)[0].getElementsByTagName('textbox');
|
|
assert.lengthOf(textboxes, 1);
|
|
assert.equal(textboxes[0].getAttribute('fieldname'), 'title');
|
|
textboxes[0].blur();
|
|
yield Zotero.Promise.delay(1);
|
|
})
|
|
|
|
it("should save an entered value when New Item is used", function* () {
|
|
var value = "Test";
|
|
var item = yield zp.newItem(Zotero.ItemTypes.getID('book'), {}, null, true);
|
|
var itemBox = doc.getElementById('zotero-editpane-item-box');
|
|
var textbox = doc.getAnonymousNodes(itemBox)[0].getElementsByTagName('textbox')[0];
|
|
textbox.value = value;
|
|
yield itemBox.blurOpenField();
|
|
item = yield Zotero.Items.getAsync(item.id);
|
|
assert.equal(item.getField('title'), value);
|
|
})
|
|
});
|
|
})
|