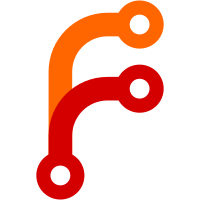
Moved schema file out of chrome and removed XML tags, since it will no longer be accessed by XMLHTTP Changed schema updater to use XPCOM components rather than XMLHTTP Converted DB class to a singleton named Scholar_DB Scholar_DB.query() now returns associative array similar to mysql_fetch_assoc() for SELECT statements rather than a mozIStorageStatementWrapper -- the mozStorage executeDataSet() function doesn't work yet, so we do it this way Added DB functions: - beginTransaction(), commitTransaction(), rollbackTransaction() - columnQuery() -- one column, multiple rows, returned as array indexed by row - getColumns() and getColumnHash DB query functions can now handle bind parameters passed as an array of object literals: e.g. [{'int':2},{'string':'foobar'}] valueQuery() now returns an int for COUNT(*) queries, so the result can be tested without a "0" being true Changed _initializeSchema to drop existing tables before creating new ones, hacked with try/catch until DROP TABLE IF EXISTS works with the mozStorage extension (it's already in the latest SQLite release) Added DB_REBUILD config flag to manually trigger schema regeneration Added debug logging at level 5 for all SQL queries Updated sample data
211 lines
9.7 KiB
SQL
211 lines
9.7 KiB
SQL
-- 2
|
|
|
|
-- IF EXISTS not yet available in available mozStorage binaries,
|
|
-- so we fake it with a bunch of try/catches in the _initializeSchema()
|
|
|
|
-- DROP TABLE IF EXISTS version;
|
|
CREATE TABLE version (
|
|
version INTEGER PRIMARY KEY
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS objects;
|
|
CREATE TABLE objects (
|
|
objectID INTEGER PRIMARY KEY,
|
|
objectTypeID INT,
|
|
title TEXT,
|
|
dateAdded DATETIME DEFAULT CURRENT_TIMESTAMP,
|
|
dateModified DATETIME DEFAULT CURRENT_TIMESTAMP,
|
|
source TEXT,
|
|
rights TEXT,
|
|
folderID INT,
|
|
orderIndex INT,
|
|
FOREIGN KEY (folderID) REFERENCES folders(folderID)
|
|
);
|
|
CREATE INDEX folderID ON objects(folderID);
|
|
|
|
-- DROP TABLE IF EXISTS objectTypes;
|
|
CREATE TABLE objectTypes (
|
|
objectTypeID INTEGER PRIMARY KEY,
|
|
typeName TEXT
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS fieldFormats;
|
|
CREATE TABLE fieldFormats (
|
|
fieldFormatID INTEGER PRIMARY KEY,
|
|
regex TEXT,
|
|
isInteger INT
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS fields;
|
|
CREATE TABLE fields (
|
|
fieldID INTEGER PRIMARY KEY,
|
|
fieldName TEXT,
|
|
fieldFormatID INT,
|
|
FOREIGN KEY (fieldFormatID) REFERENCES fieldFormat(fieldFormatID)
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS objectTypeFields;
|
|
CREATE TABLE objectTypeFields (
|
|
objectTypeID INT,
|
|
fieldID INT,
|
|
orderIndex INT,
|
|
PRIMARY KEY (objectTypeID, fieldID),
|
|
FOREIGN KEY (objectTypeID) REFERENCES objectTypes(objectTypeID),
|
|
FOREIGN KEY (fieldID) REFERENCES objectTypes(objectTypeID)
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS objectData;
|
|
CREATE TABLE objectData (
|
|
objectID INT,
|
|
fieldID INT,
|
|
value NONE,
|
|
PRIMARY KEY (objectID, fieldID),
|
|
FOREIGN KEY (objectID) REFERENCES objects(objectID),
|
|
FOREIGN KEY (fieldID) REFERENCES fields(fieldID)
|
|
);
|
|
CREATE INDEX value ON objectData (value);
|
|
|
|
-- DROP TABLE IF EXISTS keywords;
|
|
CREATE TABLE keywords (
|
|
keywordID INTEGER PRIMARY KEY,
|
|
keyword TEXT
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS objectKeywords;
|
|
CREATE TABLE objectKeywords (
|
|
objectID INT,
|
|
keywordID INT,
|
|
PRIMARY KEY (objectID, keywordID),
|
|
FOREIGN KEY (objectID) REFERENCES objects(objectID),
|
|
FOREIGN KEY (keywordID) REFERENCES keywords(keywordID)
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS creators;
|
|
CREATE TABLE creators (
|
|
creatorID INT,
|
|
creatorTypeID INT DEFAULT 1,
|
|
firstName TEXT,
|
|
lastName TEXT,
|
|
PRIMARY KEY (creatorID),
|
|
FOREIGN KEY (creatorTypeID) REFERENCES creatorTypes(creatorTypeID)
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS creatorTypes;
|
|
CREATE TABLE creatorTypes (
|
|
creatorTypeID INTEGER PRIMARY KEY,
|
|
creatorType TEXT
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS objectCreators;
|
|
CREATE TABLE objectCreators (
|
|
objectID INT,
|
|
creatorID INT,
|
|
orderIndex INT DEFAULT 0,
|
|
PRIMARY KEY (objectID, creatorID),
|
|
FOREIGN KEY (objectID) REFERENCES objects(objectID),
|
|
FOREIGN KEY (creatorID) REFERENCES creators(creatorID)
|
|
);
|
|
|
|
-- DROP TABLE IF EXISTS folders;
|
|
CREATE TABLE folders (
|
|
folderID INTEGER PRIMARY KEY,
|
|
folderName TEXT,
|
|
parentFolderID INT DEFAULT 0,
|
|
orderIndex INT,
|
|
FOREIGN KEY (parentFolderID) REFERENCES folders(folderID)
|
|
);
|
|
CREATE INDEX parentFolderID ON folders(parentFolderID);
|
|
INSERT INTO folders VALUES (0, 'root', 0, 0);
|
|
|
|
-- Some sample data
|
|
INSERT INTO objectTypes VALUES (1,'Book');
|
|
INSERT INTO objectTypes VALUES (2,'Journal Article');
|
|
|
|
INSERT INTO "fieldFormats" VALUES(1, '.*', 0);
|
|
INSERT INTO "fieldFormats" VALUES(2, '[0-9]*', 1);
|
|
INSERT INTO "fieldFormats" VALUES(3, '[0-9]{4}', 1);
|
|
|
|
INSERT INTO fields VALUES (1,'series',NULL);
|
|
INSERT INTO fields VALUES (2,'volume',NULL);
|
|
INSERT INTO fields VALUES (3,'number',NULL);
|
|
INSERT INTO fields VALUES (4,'edition',NULL);
|
|
INSERT INTO fields VALUES (5,'place',NULL);
|
|
INSERT INTO fields VALUES (6,'publisher',NULL);
|
|
INSERT INTO fields VALUES (7,'year',3);
|
|
INSERT INTO fields VALUES (8,'pages',2);
|
|
INSERT INTO fields VALUES (9,'ISBN',NULL);
|
|
INSERT INTO fields VALUES (10,'publication',NULL);
|
|
INSERT INTO fields VALUES (11,'ISSN',NULL);
|
|
|
|
INSERT INTO objectTypeFields VALUES (1,1,1);
|
|
INSERT INTO objectTypeFields VALUES (1,2,2);
|
|
INSERT INTO objectTypeFields VALUES (1,3,3);
|
|
INSERT INTO objectTypeFields VALUES (1,4,4);
|
|
INSERT INTO objectTypeFields VALUES (1,5,5);
|
|
INSERT INTO objectTypeFields VALUES (1,6,6);
|
|
INSERT INTO objectTypeFields VALUES (1,7,7);
|
|
INSERT INTO objectTypeFields VALUES (1,8,8);
|
|
INSERT INTO objectTypeFields VALUES (1,9,9);
|
|
INSERT INTO objectTypeFields VALUES (2,10,1);
|
|
INSERT INTO objectTypeFields VALUES (2,2,2);
|
|
INSERT INTO objectTypeFields VALUES (2,3,3);
|
|
INSERT INTO objectTypeFields VALUES (2,8,4);
|
|
|
|
INSERT INTO "objects" VALUES(1, 1, 'Online connections: Internet interpersonal relationships', '2006-03-12 05:24:40', '2006-03-12 05:24:40', NULL, NULL, 0, 5);
|
|
INSERT INTO "objects" VALUES(2, 1, 'Computer-Mediated Communication: Human-to-Human Communication Across the Internet', '2006-03-12 05:25:50', '2006-03-12 05:25:50', NULL, NULL, 0, 2);
|
|
INSERT INTO "objects" VALUES(3, 2, 'Residential propinquity as a factor in marriage selection', '2006-03-12 05:26:37', '2006-03-12 05:26:37', NULL, NULL, 0, 1);
|
|
INSERT INTO "objects" VALUES(4, 1, 'Connecting: how we form social bonds and communities in the Internet age', '2006-03-12 05:27:15', '2006-03-12 05:27:15', NULL, NULL, 0, 4);
|
|
INSERT INTO "objects" VALUES(5, 1, 'Male, Female, Email: The Struggle for Relatedness in a Paranoid Society', '2006-03-12 05:27:36', '2006-03-12 05:27:36', NULL, NULL, 0, 3);
|
|
INSERT INTO "objects" VALUES(6, 2, 'Social Implications of Sociology', '2006-03-12 05:27:53', '2006-03-12 05:27:53', NULL, NULL, 0, 7);
|
|
INSERT INTO "objects" VALUES(7, 1, 'Social Pressures in Informal Groups: A Study of Human Factors in Housing', '2006-03-12 05:28:05', '2006-03-12 05:28:05', NULL, NULL, 0, 6);
|
|
INSERT INTO "objects" VALUES(8, 1, 'Cybersociety 2.0: Revisiting Computer-Mediated Community and Technology', '2006-03-12 05:28:37', '2006-03-12 05:28:37', NULL, NULL, 0, 9);
|
|
INSERT INTO "objects" VALUES(9, 2, 'The Computer as a Communication Device', '2006-03-12 05:29:03', '2006-03-12 05:29:03', NULL, NULL, 0, 8);
|
|
INSERT INTO "objects" VALUES(10, 2, 'What Does Research Say about the Nature of Computer-mediated Communication: Task-Oriented, Social-Emotion-Oriented, or Both?', '2006-03-12 05:29:12', '2006-03-12 05:29:12', NULL, NULL, 0, 13);
|
|
INSERT INTO "objects" VALUES(11, 1, 'The second self: computers and the human spirit', '2006-03-12 05:30:38', '2006-03-12 05:30:38', NULL, NULL, 0, 10);
|
|
INSERT INTO "objects" VALUES(12, 1, 'Life on the screen: identity in the age of the Internet', '2006-03-12 05:30:49', '2006-03-12 05:30:49', NULL, NULL, 0, 11);
|
|
INSERT INTO "objects" VALUES(13, 2, 'The computer conference: An altered state of communication', '2006-03-12 05:31:00', '2006-03-12 05:31:00', NULL, NULL, 0, 12);
|
|
INSERT INTO "objects" VALUES(14, 2, 'Computer Networks as Social Networks: Collaborative Work, Telework, and Community', '2006-03-12 05:31:17', '2006-03-12 05:31:17', NULL, NULL, 0, 14);
|
|
INSERT INTO "objects" VALUES(15, 1, 'The Internet in everyday life', '2006-03-12 05:31:41', '2006-03-12 05:31:41', NULL, NULL, 0, 15);
|
|
|
|
INSERT INTO "objectData" VALUES(1, 7, 2001);
|
|
INSERT INTO "objectData" VALUES(1, 5, 'Cresskill, N.J.');
|
|
INSERT INTO "objectData" VALUES(1, 6, 'Hampton Press');
|
|
INSERT INTO "objectData" VALUES(2, 7, 2002);
|
|
INSERT INTO "objectData" VALUES(2, 6, 'Allyn & Bacon Publishers');
|
|
INSERT INTO "objectData" VALUES(2, 8, 347);
|
|
INSERT INTO "objectData" VALUES(2, 9, '0-205-32145-3');
|
|
|
|
INSERT INTO "creators" VALUES(1, 1, 'Susan B.', 'Barnes');
|
|
INSERT INTO "creators" VALUES(2, 1, 'J.S.', 'Bassard');
|
|
INSERT INTO "creators" VALUES(3, 1, 'Mary', 'Chayko');
|
|
INSERT INTO "creators" VALUES(4, 1, 'Michael', 'Civin');
|
|
INSERT INTO "creators" VALUES(5, 1, 'Paul', 'DiMaggio');
|
|
INSERT INTO "creators" VALUES(6, 1, 'Leon', 'Festinger');
|
|
INSERT INTO "creators" VALUES(7, 1, 'Stanley', 'Schachter');
|
|
INSERT INTO "creators" VALUES(8, 1, 'Kurt', 'Back');
|
|
INSERT INTO "creators" VALUES(9, 1, 'Steven G.', 'Jones');
|
|
INSERT INTO "creators" VALUES(10, 1, 'J.C.R.', 'Licklider');
|
|
INSERT INTO "creators" VALUES(11, 1, 'Robert W.', 'Taylor');
|
|
INSERT INTO "creators" VALUES(12, 1, 'Yuliang', 'Lui');
|
|
INSERT INTO "creators" VALUES(13, 1, 'Sherry', 'Turkle');
|
|
INSERT INTO "creators" VALUES(14, 1, 'J.', 'Vallee');
|
|
INSERT INTO "creators" VALUES(15, 1, 'Barry', 'Wellman');
|
|
|
|
INSERT INTO "objectCreators" VALUES(1, 1, 0);
|
|
INSERT INTO "objectCreators" VALUES(2, 1, 0);
|
|
INSERT INTO "objectCreators" VALUES(3, 2, 0);
|
|
INSERT INTO "objectCreators" VALUES(4, 3, 0);
|
|
INSERT INTO "objectCreators" VALUES(5, 4, 0);
|
|
INSERT INTO "objectCreators" VALUES(6, 5, 0);
|
|
INSERT INTO "objectCreators" VALUES(7, 6, 0);
|
|
INSERT INTO "objectCreators" VALUES(8, 9, 0);
|
|
INSERT INTO "objectCreators" VALUES(9, 10, 0);
|
|
INSERT INTO "objectCreators" VALUES(10, 12, 0);
|
|
INSERT INTO "objectCreators" VALUES(11, 13, 0);
|
|
INSERT INTO "objectCreators" VALUES(12, 13, 0);
|
|
INSERT INTO "objectCreators" VALUES(13, 14, 0);
|
|
INSERT INTO "objectCreators" VALUES(14, 15, 0);
|
|
INSERT INTO "objectCreators" VALUES(15, 15, 0);
|
|
INSERT INTO "objectCreators" VALUES(7, 7, 1);
|
|
INSERT INTO "objectCreators" VALUES(7, 8, 2);
|
|
INSERT INTO "objectCreators" VALUES(9, 11, 1);
|