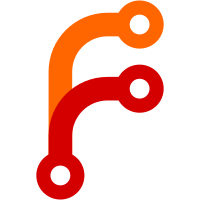
Apologies for the massive (and, due to data_access.js splitting, difficult-to-follow) commit. Please note that external code that accesses the data layer may need to be tweaked for compatibility. Here's a comprehensive-as-possible changelog: - Added server sync functionality (incomplete) - Overhaul of data layer - Split data_access.js into separate files (item.js, items.js, creator.js, etc.) - Made creators and collections first-class objects, similar to items - Constructors now take id as first parameter, e.g. new Zotero.Item(1234, 'book'), to allow explicit id setting and id changing - Made various data layer operations (including attachment fields) require a save() rather than making direct DB changes - Better handling of unsaved objects - Item.setCreator() now takes creator objects instead of creator ids, and Item.save() will auto-save unsaved creators - clone() now works on unsaved objects - Newly created object instances are now disabled after save() to force refetch of globally accessible instance using Zotero.(Items|Creators|etc.).get() - Added secondary lookup key to data objects - Deprecated getID() and getItemType() methods in favor of .id and .itemTypeID properties - toArray() deprecated in favor of serialize(), which has a somewhat modified format - Added support for multiple creators with identical data -- currently unimplemented in interface and most of data layer - Added Item.diff() for comparing item metadata - Database changes - Added SQLite triggers to enforce foreign key constraints - Added Zotero.DB.transactionVacuum flag to run a VACUUM after a transaction - Added Zotero.DB.transactionDate, .transactionDateTime, and transactionTimestamp to retrieve consistent timestamps for entire transaction - Properly store 64-bit integers - Set PRAGMA locking_mode=EXCLUSIVE on database - Set SQLite page size to 4096 on new databases - Set SQLite page cache to 8MB - Do some database cleanup and integrity checking on migration from 1.0 branch - Removed IF NOT EXISTS from userdata.sql CREATE statements -- userdata.sql is now processed only on DB initialization - Removed itemNoteTitles table and moved titles into itemNotes - Abstracted metadata edit box and note box into flexible XBL bindings with various modes, including read-only states - Massive speed-up of item tree view - Several fixes from 1.0 branch for Fx3 compatibility - Added Notifier observer to log delete events for syncing - Zotero.Utilities changes - New methods getSQLDataType() and md5() - Removed onError from Zotero.Utilities.HTTP.doGet() - Don't display more than 1024 characters in doPost() debug output - Don't display passwords in doPost() debug output - Added Zotero.Notifier.untrigger() -- currently unused - Added Zotero.reloadDataObjects() to reset all in-memory objects - Added |chars| parameter to Zotero.randomString(len, chars) - Added Zotero.Date.getUnixTimestamp() and Date.toUnixTimestamp(JSDate) - Adjusted zotero-service.js to simplify file inclusion Various things (such as tags) are temporarily broken.
151 lines
4.2 KiB
JavaScript
151 lines
4.2 KiB
JavaScript
const ZOTERO_CONTRACTID = '@zotero.org/Zotero;1';
|
|
const ZOTERO_CLASSNAME = 'Zotero';
|
|
const ZOTERO_CID = Components.ID('{e4c61080-ec2d-11da-8ad9-0800200c9a66}');
|
|
const ZOTERO_IID = Components.interfaces.chnmIZoteroService; //unused
|
|
|
|
const Cc = Components.classes;
|
|
const Ci = Components.interfaces;
|
|
|
|
// Assign the global scope to a variable to passed via wrappedJSObject
|
|
var ZoteroWrapped = this;
|
|
|
|
|
|
/********************************************************************
|
|
* Include the core objects to be stored within XPCOM
|
|
*********************************************************************/
|
|
|
|
var xpcomFiles = [ 'zotero',
|
|
'annotate', 'attachments', 'cite', 'cite_compat', 'collectionTreeView',
|
|
'data_access', 'data/item', 'data/items', 'data/collection', 'data/collections',
|
|
'data/cachedTypes', 'data/creator', 'data/creators', 'data/itemFields',
|
|
'data/notes', 'data/tags', 'db', 'file', 'fulltext', 'id', 'ingester', 'integration',
|
|
'itemTreeView', 'mime', 'notifier', 'progressWindow', 'quickCopy', 'report',
|
|
'schema', 'search', 'sync', 'timeline', 'translate', 'utilities'];
|
|
|
|
for (var i=0; i<xpcomFiles.length; i++) {
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://zotero/content/xpcom/" + xpcomFiles[i] + ".js");
|
|
}
|
|
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://global/content/nsTransferable.js");
|
|
|
|
Cc["@mozilla.org/moz/jssubscript-loader;1"]
|
|
.getService(Ci.mozIJSSubScriptLoader)
|
|
.loadSubScript("chrome://global/content/nsDragAndDrop.js");
|
|
|
|
/********************************************************************/
|
|
|
|
|
|
// Initialize the Zotero service
|
|
//
|
|
// This runs when ZoteroService is first requested.
|
|
// Calls to other XPCOM components must be in here rather than in top-level
|
|
// code, as other components may not have yet been initialized.
|
|
function setupService(){
|
|
Zotero.init();
|
|
}
|
|
|
|
function ZoteroService(){
|
|
this.wrappedJSObject = ZoteroWrapped.Zotero;
|
|
setupService();
|
|
}
|
|
|
|
|
|
/**
|
|
* Convenience method to replicate window.alert()
|
|
**/
|
|
function alert(msg){
|
|
Cc["@mozilla.org/embedcomp/prompt-service;1"]
|
|
.getService(Ci.nsIPromptService)
|
|
.alert(null, "", msg);
|
|
}
|
|
|
|
/**
|
|
* Convenience method to replicate window.confirm()
|
|
**/
|
|
function confirm(msg){
|
|
return Cc["@mozilla.org/embedcomp/prompt-service;1"]
|
|
.getService(Ci.nsIPromptService)
|
|
.confirm(null, "", msg);
|
|
}
|
|
|
|
|
|
/**
|
|
* Convenience method to replicate window.setTimeout()
|
|
**/
|
|
function setTimeout(func, ms){
|
|
var timer = Components.classes["@mozilla.org/timer;1"].
|
|
createInstance(Components.interfaces.nsITimer);
|
|
// {} implements nsITimerCallback
|
|
timer.initWithCallback({notify:func}, ms,
|
|
Components.interfaces.nsITimer.TYPE_ONE_SHOT);
|
|
}
|
|
|
|
|
|
//
|
|
// XPCOM goop
|
|
//
|
|
ZoteroService.prototype = {
|
|
QueryInterface: function(iid){
|
|
if (!iid.equals(Components.interfaces.nsISupports) &&
|
|
!iid.equals(ZOTERO_IID)){ // interface unused
|
|
throw Components.results.NS_ERROR_NO_INTERFACE;
|
|
}
|
|
return this;
|
|
}
|
|
};
|
|
|
|
|
|
var ZoteroFactory = {
|
|
createInstance: function(outer, iid){
|
|
if (outer != null){
|
|
throw Components.results.NS_ERROR_NO_AGGREGATION;
|
|
}
|
|
return new ZoteroService().QueryInterface(iid);
|
|
}
|
|
};
|
|
|
|
|
|
var ZoteroModule = {
|
|
_firstTime: true,
|
|
|
|
registerSelf: function(compMgr, fileSpec, location, type){
|
|
if (!this._firstTime){
|
|
throw Components.results.NS_ERROR_FACTORY_REGISTER_AGAIN;
|
|
}
|
|
this._firstTime = false;
|
|
|
|
compMgr =
|
|
compMgr.QueryInterface(Components.interfaces.nsIComponentRegistrar);
|
|
|
|
compMgr.registerFactoryLocation(ZOTERO_CID,
|
|
ZOTERO_CLASSNAME,
|
|
ZOTERO_CONTRACTID,
|
|
fileSpec,
|
|
location,
|
|
type);
|
|
},
|
|
|
|
unregisterSelf: function(compMgr, location, type){
|
|
compMgr =
|
|
compMgr.QueryInterface(Components.interfaces.nsIComponentRegistrar);
|
|
compMgr.unregisterFactoryLocation(ZOTERO_CID, location);
|
|
},
|
|
|
|
getClassObject: function(compMgr, cid, iid){
|
|
if (!cid.equals(ZOTERO_CID)){
|
|
throw Components.results.NS_ERROR_NO_INTERFACE;
|
|
}
|
|
if (!iid.equals(Components.interfaces.nsIFactory)){
|
|
throw Components.results.NS_ERROR_NOT_IMPLEMENTED;
|
|
}
|
|
return ZoteroFactory;
|
|
},
|
|
|
|
canUnload: function(compMgr){ return true; }
|
|
};
|
|
|
|
function NSGetModule(comMgr, fileSpec){ return ZoteroModule; }
|