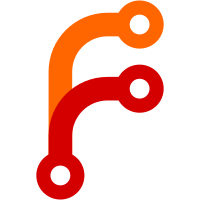
- Moved ::_get() and _set() from Collection/Search into DataObject, and disabled in Item - Don't disable new items after save. We now put new objects into the DataObjects cache from save() so that changes made post-save are picked up by other code using .get(). - Added 'skipCache' save() option to avoid reloading data on new objects and adding them to the cache. (This will be used in syncing, where objects might be in another library where they're not needed right away.) Objects created with this option are instead disabled to prevent reuse. - Modified some tests to try to make sure we're reloading everything properly after a save. - Documented save() options
56 lines
1.4 KiB
JavaScript
56 lines
1.4 KiB
JavaScript
describe("Item pane", function () {
|
|
var win, doc, itemsView;
|
|
|
|
before(function* () {
|
|
win = yield loadZoteroPane();
|
|
doc = win.document;
|
|
itemsView = win.ZoteroPane.itemsView;
|
|
});
|
|
after(function () {
|
|
win.close();
|
|
});
|
|
|
|
describe("Info pane", function () {
|
|
it("should refresh on item update", function* () {
|
|
var item = new Zotero.Item('book');
|
|
var id = yield item.saveTx();
|
|
|
|
var itemBox = doc.getElementById('zotero-editpane-item-box');
|
|
var label = doc.getAnonymousNodes(itemBox)[0].getElementsByAttribute('fieldname', 'title')[1];
|
|
assert.equal(label.textContent, '');
|
|
|
|
item.setField('title', 'Test');
|
|
yield item.saveTx();
|
|
|
|
var label = doc.getAnonymousNodes(itemBox)[0].getElementsByAttribute('fieldname', 'title')[1];
|
|
assert.equal(label.textContent, 'Test');
|
|
|
|
yield Zotero.Items.erase(id);
|
|
})
|
|
})
|
|
|
|
describe("Note pane", function () {
|
|
it("should refresh on note update", function* () {
|
|
var item = new Zotero.Item('note');
|
|
var id = yield item.saveTx();
|
|
|
|
// Wait for the editor
|
|
var noteBox = doc.getElementById('zotero-note-editor');
|
|
var val = false;
|
|
do {
|
|
try {
|
|
val = noteBox.noteField.value;
|
|
}
|
|
catch (e) {}
|
|
yield Zotero.Promise.delay(1);
|
|
}
|
|
while (val === false)
|
|
assert.equal(noteBox.noteField.value, '');
|
|
|
|
item.setNote('<p>Test</p>');
|
|
yield item.saveTx();
|
|
|
|
assert.equal(noteBox.noteField.value, '<p>Test</p>');
|
|
})
|
|
})
|
|
})
|