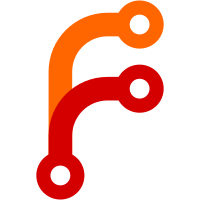
Relations are now properties of collections and items rather than first-class objects, stored in separate collectionRelations and itemRelations tables with ids for subjects, with foreign keys to the associated data objects. Related items now use dc:relation relations rather than a separate table (among other reasons, because API syncing won't necessarily sync both items at the same time, so they can't be stored by id). The UI assigns related-item relations bidirectionally, and checks for related-item and linked-object relations are done unidirectionally by default. dc:isReplacedBy is now dc:replaces, so that the subject is an existing object, and the predicate is now named Zotero.Attachments.replacedItemPredicate. Some additional work is still needed, notably around following replaced-item relations, and migration needs to be tested more fully, but this seems to mostly work.
103 lines
3 KiB
JavaScript
103 lines
3 KiB
JavaScript
"use strict";
|
|
|
|
describe("Related Box", function () {
|
|
var win, doc, itemsView;
|
|
|
|
before(function* () {
|
|
win = yield loadZoteroPane();
|
|
doc = win.document;
|
|
itemsView = win.ZoteroPane.itemsView;
|
|
});
|
|
after(function () {
|
|
win.close();
|
|
})
|
|
|
|
describe("Add button", function () {
|
|
it("should add a related item", function* () {
|
|
var item1 = yield createDataObject('item');
|
|
var item2 = yield createDataObject('item');
|
|
|
|
// Select the Related pane
|
|
var tabbox = doc.getElementById('zotero-view-tabbox');
|
|
tabbox.selectedIndex = 3;
|
|
var relatedbox = doc.getElementById('zotero-editpane-related');
|
|
assert.lengthOf(relatedbox.id('relatedRows').childNodes, 0);
|
|
|
|
// Click the Add button to open the Select Items dialog
|
|
setTimeout(function () {
|
|
relatedbox.id('addButton').click();
|
|
});
|
|
var selectWin = yield waitForWindow('chrome://zotero/content/selectItemsDialog.xul');
|
|
// wrappedJSObject isn't working on zotero-collections-tree for some reason, so
|
|
// just wait for the items tree to be created and select it directly
|
|
do {
|
|
var view = selectWin.document.getElementById('zotero-items-tree').view.wrappedJSObject;
|
|
yield Zotero.Promise.delay(50);
|
|
}
|
|
while (!view);
|
|
var deferred = Zotero.Promise.defer();
|
|
view.addEventListener('load', () => deferred.resolve());
|
|
yield deferred.promise;
|
|
|
|
// Select the other item
|
|
for (let i = 0; i < view.rowCount; i++) {
|
|
if (view.getRow(i).ref.id == item1.id) {
|
|
view.selection.select(i);
|
|
}
|
|
}
|
|
selectWin.document.documentElement.acceptDialog();
|
|
|
|
// Wait for relations list to populate
|
|
do {
|
|
yield Zotero.Promise.delay(50);
|
|
}
|
|
while (!relatedbox.id('relatedRows').childNodes.length);
|
|
|
|
assert.lengthOf(relatedbox.id('relatedRows').childNodes, 1);
|
|
|
|
var items = item1.relatedItems;
|
|
assert.lengthOf(items, 1);
|
|
assert.equal(items[0], item2.key);
|
|
|
|
// Relation should be assigned bidirectionally
|
|
var items = item2.relatedItems;
|
|
assert.lengthOf(items, 1);
|
|
assert.equal(items[0], item1.key);
|
|
})
|
|
})
|
|
|
|
describe("Remove button", function () {
|
|
it("should remove a related item", function* () {
|
|
var item1 = yield createDataObject('item');
|
|
var item2 = yield createDataObject('item');
|
|
|
|
yield item1.loadRelations();
|
|
item1.addRelatedItem(item2);
|
|
yield item1.save();
|
|
yield item2.loadRelations();
|
|
item2.addRelatedItem(item1);
|
|
yield item2.save();
|
|
|
|
// Select the Related pane
|
|
var tabbox = doc.getElementById('zotero-view-tabbox');
|
|
tabbox.selectedIndex = 3;
|
|
var relatedbox = doc.getElementById('zotero-editpane-related');
|
|
|
|
// Wait for relations list to populate
|
|
do {
|
|
yield Zotero.Promise.delay(50);
|
|
}
|
|
while (!relatedbox.id('relatedRows').childNodes.length);
|
|
|
|
doc.getAnonymousNodes(relatedbox)[0]
|
|
.getElementsByAttribute('value', '-')[0]
|
|
.click();
|
|
|
|
// Wait for relations list to clear
|
|
do {
|
|
yield Zotero.Promise.delay(50);
|
|
}
|
|
while (relatedbox.id('relatedRows').childNodes.length);
|
|
})
|
|
})
|
|
})
|