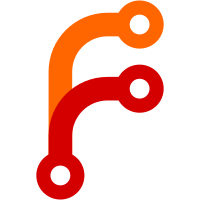
This changes `createDataObject()` to pass `skipSelect: true` for objects other than items. If a test is creating a bunch of collections, there's no reason for each one to be selected and for an items list to start to load. If a test does need a new collection or search to be selected, it can call the new convenience function `await select(win, obj)`, which will select the passed object in the collection tree and wait for its items list to load. I'm hoping this reduces random test failures due to items list churn.
45 lines
1.3 KiB
JavaScript
45 lines
1.3 KiB
JavaScript
"use strict";
|
|
|
|
describe("Zotero.LibraryTree", function() {
|
|
var win, zp, cv, itemsView;
|
|
|
|
// Load Zotero pane and select library
|
|
before(function* () {
|
|
win = yield loadZoteroPane();
|
|
zp = win.ZoteroPane;
|
|
cv = zp.collectionsView;
|
|
});
|
|
beforeEach(function* () {
|
|
yield selectLibrary(win);
|
|
itemsView = zp.itemsView;
|
|
})
|
|
after(function () {
|
|
win.close();
|
|
});
|
|
|
|
describe("#getRowIndexByID()", function () {
|
|
it("should return the row index of an item", async function () {
|
|
var collection = await createDataObject('collection');
|
|
await select(win, collection);
|
|
var item = await createDataObject('item', { collections: [collection.id] });
|
|
var view = zp.itemsView;
|
|
assert.strictEqual(view.getRowIndexByID(item.treeViewID), 0);
|
|
});
|
|
});
|
|
|
|
describe("#_removeRow()", function () {
|
|
it("should remove the last row", async function () {
|
|
var collection = await createDataObject('collection');
|
|
await select(win, collection);
|
|
await createDataObject('item', { collections: [collection.id] });
|
|
await createDataObject('item', { collections: [collection.id] });
|
|
|
|
var view = zp.itemsView;
|
|
var treeViewID = view.getRow(1).id;
|
|
zp.itemsView._removeRow(1);
|
|
|
|
assert.equal(view.rowCount, 1);
|
|
assert.isFalse(view.getRowIndexByID(treeViewID));
|
|
});
|
|
});
|
|
})
|