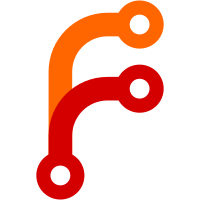
When an export translator is selected for Quick Copy, Quick Copy initialization triggers translator initialization a few seconds after startup, because the translator code needs to be available synchronously for drag/drop. A Quick Copy test was changing the setting to BibTeX, which was resulting in random timeouts after subsequent resetDB() calls due to slow translator loading. This change skips initialization in test mode. This might actually fix a lot of timeouts on Travis in the second half of the tests... This also resets the Quick Copy pref in those tests so that it's left at the default, though really we should automatically reset all prefs after all test groups and in resetDB().
76 lines
2.4 KiB
JavaScript
76 lines
2.4 KiB
JavaScript
describe("Zotero.QuickCopy", function() {
|
|
var quickCopyPref;
|
|
var prefName = "export.quickCopy.setting";
|
|
|
|
before(function () {
|
|
Zotero.Prefs.clear(prefName);
|
|
quickCopyPref = Zotero.Prefs.get(prefName);
|
|
quickCopyPref = JSON.stringify(Zotero.QuickCopy.unserializeSetting(quickCopyPref));
|
|
});
|
|
|
|
afterEach(function () {
|
|
Zotero.Prefs.clear(prefName);
|
|
});
|
|
|
|
// TODO: These should set site-specific prefs and test the actual response against it,
|
|
// but that will need to wait for 5.0. For now, just make sure they don't fail.
|
|
describe("#getFormatFromURL()", function () {
|
|
it("should handle an HTTP URL", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('http://foo.com/'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle an HTTPS URL", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('https://foo.com/'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle a domain and path", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('http://foo.com/bar'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle a local host", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('http://foo/'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle a domain with a trailing period", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('http://foo.com.'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle an about: URL", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('about:blank'), quickCopyPref);
|
|
})
|
|
|
|
it("should handle a chrome URL", function () {
|
|
assert.deepEqual(Zotero.QuickCopy.getFormatFromURL('chrome://zotero/content/tab.xul'), quickCopyPref);
|
|
})
|
|
})
|
|
|
|
describe("#getContentFromItems()", function () {
|
|
it("should generate BibTeX", function* () {
|
|
var item = yield createDataObject('item');
|
|
var content = "";
|
|
var worked = false;
|
|
|
|
yield Zotero.Translators.init();
|
|
|
|
var translatorID = '9cb70025-a888-4a29-a210-93ec52da40d4'; // BibTeX
|
|
var format = 'export=' + translatorID;
|
|
Zotero.Prefs.set(prefName, format);
|
|
// Translator code for selected format is loaded automatically, so wait for it
|
|
var translator = Zotero.Translators.get(translatorID);
|
|
while (!translator.code) {
|
|
yield Zotero.Promise.delay(50);
|
|
}
|
|
|
|
Zotero.QuickCopy.getContentFromItems(
|
|
[item],
|
|
format,
|
|
(obj, w) => {
|
|
content = obj.string;
|
|
worked = w;
|
|
}
|
|
);
|
|
assert.isTrue(worked);
|
|
assert.isTrue(content.trim().startsWith('@'));
|
|
});
|
|
});
|
|
})
|