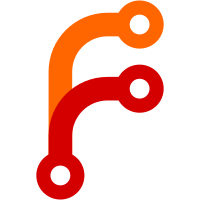
* Starting to work through lint errors * libsignal-protocol: Update changes for primary repo compatibility * Step 1: task_with_timeout rename * Step 2: Apply the changes to TaskWithTimeout.ts * Step 1: All to-be-converted libtextsecure/*.js files moved * Step 2: No Typescript errors! * Get libtextsecure tests passing again * TSLint errors down to 1 * Compilation succeeds, no lint errors or test failures * WebSocketResources - update import for case-sensitive filesystems * Fixes for lint-deps * Remove unnecessary @ts-ignore * Fix inability to message your own contact after link * Add log message for the end of migration 20 * lint fix
49 lines
1.1 KiB
TypeScript
49 lines
1.1 KiB
TypeScript
// tslint:disable no-default-export
|
|
|
|
import utils from './Helpers';
|
|
|
|
// Default implmentation working with localStorage
|
|
const localStorageImpl = {
|
|
put(key: string, value: any) {
|
|
if (value === undefined) {
|
|
throw new Error('Tried to store undefined');
|
|
}
|
|
localStorage.setItem(`${key}`, utils.jsonThing(value));
|
|
},
|
|
|
|
get(key: string, defaultValue: any) {
|
|
const value = localStorage.getItem(`${key}`);
|
|
if (value === null) {
|
|
return defaultValue;
|
|
}
|
|
return JSON.parse(value);
|
|
},
|
|
|
|
remove(key: string) {
|
|
localStorage.removeItem(`${key}`);
|
|
},
|
|
};
|
|
|
|
export interface StorageInterface {
|
|
put(key: string, value: any): void | Promise<void>;
|
|
get(key: string, defaultValue: any): any;
|
|
remove(key: string): void | Promise<void>;
|
|
}
|
|
|
|
const Storage = {
|
|
impl: localStorageImpl as StorageInterface,
|
|
|
|
put(key: string, value: any) {
|
|
return Storage.impl.put(key, value);
|
|
},
|
|
|
|
get(key: string, defaultValue: any) {
|
|
return Storage.impl.get(key, defaultValue);
|
|
},
|
|
|
|
remove(key: string) {
|
|
return Storage.impl.remove(key);
|
|
},
|
|
};
|
|
|
|
export default Storage;
|