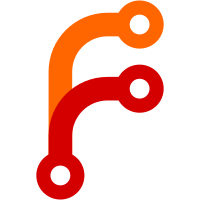
Biggest changes forced by this: alt tags for all images, resulting in new strings added to messages.json, and a new i18n paramter/prop added in a plot of places. Another change of note is that there are two new tslint.json files under ts/test and ts/styleguide to relax our rules a bit there. This required a change to our package.json script, as manually specifying the config file there made it ignore our tslint.json files in subdirectories
48 lines
1.5 KiB
TypeScript
48 lines
1.5 KiB
TypeScript
import React from 'react';
|
|
|
|
import { getSizeClass } from '../../util/emoji';
|
|
import { Emojify } from './Emojify';
|
|
import { AddNewLines } from './AddNewLines';
|
|
import { Linkify } from './Linkify';
|
|
|
|
import { Localizer, RenderTextCallback } from '../../types/Util';
|
|
|
|
interface Props {
|
|
text: string;
|
|
/** If set, all emoji will be the same size. Otherwise, just one emoji will be large. */
|
|
disableJumbomoji?: boolean;
|
|
/** If set, links will be left alone instead of turned into clickable `<a>` tags. */
|
|
disableLinks?: boolean;
|
|
i18n: Localizer;
|
|
}
|
|
|
|
const renderNewLines: RenderTextCallback = ({
|
|
text: textWithNewLines,
|
|
key,
|
|
}) => <AddNewLines key={key} text={textWithNewLines} />;
|
|
|
|
const renderLinks: RenderTextCallback = ({ text: textWithLinks, key }) => (
|
|
<Linkify key={key} text={textWithLinks} renderNonLink={renderNewLines} />
|
|
);
|
|
|
|
/**
|
|
* This component makes it very easy to use all three of our message formatting
|
|
* components: `Emojify`, `Linkify`, and `AddNewLines`. Because each of them is fully
|
|
* configurable with their `renderXXX` props, this component will assemble all three of
|
|
* them for you.
|
|
*/
|
|
export class MessageBody extends React.Component<Props> {
|
|
public render() {
|
|
const { text, disableJumbomoji, disableLinks, i18n } = this.props;
|
|
const sizeClass = disableJumbomoji ? '' : getSizeClass(text);
|
|
|
|
return (
|
|
<Emojify
|
|
text={text}
|
|
sizeClass={sizeClass}
|
|
renderNonEmoji={disableLinks ? renderNewLines : renderLinks}
|
|
i18n={i18n}
|
|
/>
|
|
);
|
|
}
|
|
}
|