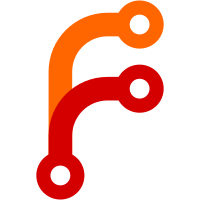
* Add certificate pinning on https service requests Make https requests to the server using node apis instead of browser apis, so we can specify our own CA list, which contains only our own CA. This protects us from MITM by a rogue CA. As a bonus, this let's us drop the use of non-standard ports and just use good ol' default 443 all the time, at least for http requests. // FREEBIE * Make certificateAuthorities an option on requests Modify node-based xhr implementation based on driverdan/node-XMLHttpRequest, adding support for setting certificate authorities on each request. This allows us to pin our master CA for requests to the server and cdn but not to the s3 attachment server, for instance. Also fix an exception when sending binary data in a request: it is submitted as an array buffer, and must be converted to a node Buffer since we are now using a node based request api. // FREEBIE * Import node-based xhr implementation Add a copy of https://github.com/driverdan/node-XMLHttpRequest@86ff70e, and expose it to the renderer in the preload script. In later commits this module will be extended to support custom certificate authorities. // FREEBIE * Support "arraybuffer" responseType on requests When fetching attachments, we want the result as binary data rather than a utf8 string. This lets our node-based XMLHttpRequest honor the responseType property if it is set on the xhr. Note that naively using the raw `.buffer` from a node Buffer won't work, since it is a reuseable backing buffer that is often much larger than the actual content defined by the Buffer's offset and length. Instead, we'll prepare a return buffer based on the response's content length header, and incrementally write chunks of data into it as they arrive. // FREEBIE * Switch to self-signed server endpoint * Log more error info on failed requests With the node-based xhr, relevant error info are stored in statusText and responseText when a request fails. // FREEBIE * Add node-based websocket w/ support for custom CA // FREEBIE * Support handling array buffers instead of blobs Our node-based websocket calls onmessage with an arraybuffer instead of a blob. For robustness (on the off chance we switch or update the socket implementation agian) I've kept the machinery for converting blobs to array buffers. // FREEBIE * Destroy all wacky server ports // FREEBIE
573 lines
19 KiB
JavaScript
573 lines
19 KiB
JavaScript
/*
|
|
* vim: ts=4:sw=4:expandtab
|
|
*/
|
|
|
|
;(function() {
|
|
'use strict';
|
|
window.onInvalidStateError = function(e) {
|
|
console.log(e);
|
|
};
|
|
|
|
console.log('background page reloaded');
|
|
console.log('environment:', window.config.environment);
|
|
|
|
var initialLoadComplete = false;
|
|
window.owsDesktopApp = {};
|
|
|
|
// start a background worker for ecc
|
|
textsecure.startWorker('js/libsignal-protocol-worker.js');
|
|
Whisper.KeyChangeListener.init(textsecure.storage.protocol);
|
|
textsecure.storage.protocol.on('removePreKey', function() {
|
|
getAccountManager().refreshPreKeys();
|
|
});
|
|
|
|
var SERVER_URL = window.config.serverUrl;
|
|
var messageReceiver;
|
|
window.getSocketStatus = function() {
|
|
if (messageReceiver) {
|
|
return messageReceiver.getStatus();
|
|
} else {
|
|
return -1;
|
|
}
|
|
};
|
|
Whisper.events = _.clone(Backbone.Events);
|
|
var accountManager;
|
|
window.getAccountManager = function() {
|
|
if (!accountManager) {
|
|
var USERNAME = storage.get('number_id');
|
|
var PASSWORD = storage.get('password');
|
|
accountManager = new textsecure.AccountManager(
|
|
SERVER_URL, USERNAME, PASSWORD
|
|
);
|
|
accountManager.addEventListener('registration', function() {
|
|
if (!Whisper.Registration.everDone()) {
|
|
storage.put('safety-numbers-approval', false);
|
|
}
|
|
Whisper.Registration.markDone();
|
|
console.log("dispatching registration event");
|
|
Whisper.events.trigger('registration_done');
|
|
});
|
|
}
|
|
return accountManager;
|
|
};
|
|
|
|
storage.fetch();
|
|
|
|
// We need this 'first' check because we don't want to start the app up any other time
|
|
// than the first time. And storage.fetch() will cause onready() to fire.
|
|
var first = true;
|
|
storage.onready(function() {
|
|
if (!first) {
|
|
return;
|
|
}
|
|
first = false;
|
|
|
|
start();
|
|
});
|
|
|
|
window.getSyncRequest = function() {
|
|
return new textsecure.SyncRequest(textsecure.messaging, messageReceiver);
|
|
};
|
|
|
|
Whisper.events.on('shutdown', function() {
|
|
if (messageReceiver) {
|
|
messageReceiver.close().then(function() {
|
|
messageReceiver = null;
|
|
Whisper.events.trigger('shutdown-complete');
|
|
});
|
|
} else {
|
|
Whisper.events.trigger('shutdown-complete');
|
|
}
|
|
});
|
|
|
|
function start() {
|
|
ConversationController.load();
|
|
|
|
window.dispatchEvent(new Event('storage_ready'));
|
|
|
|
console.log("listening for registration events");
|
|
Whisper.events.on('registration_done', function() {
|
|
console.log("handling registration event");
|
|
connect(true);
|
|
});
|
|
|
|
var appView = window.owsDesktopApp.appView = new Whisper.AppView({el: $('body')});
|
|
|
|
Whisper.WallClockListener.init(Whisper.events);
|
|
Whisper.RotateSignedPreKeyListener.init(Whisper.events);
|
|
Whisper.ExpiringMessagesListener.init(Whisper.events);
|
|
|
|
if (Whisper.Import.isIncomplete()) {
|
|
console.log('Import was interrupted, showing import error screen');
|
|
appView.openImporter();
|
|
} else if (Whisper.Registration.everDone()) {
|
|
connect();
|
|
appView.openInbox({
|
|
initialLoadComplete: initialLoadComplete
|
|
});
|
|
} else {
|
|
appView.openInstallChoice();
|
|
}
|
|
|
|
Whisper.events.on('showDebugLog', function() {
|
|
appView.openDebugLog();
|
|
});
|
|
Whisper.events.on('unauthorized', function() {
|
|
appView.inboxView.networkStatusView.update();
|
|
});
|
|
Whisper.events.on('reconnectTimer', function() {
|
|
appView.inboxView.networkStatusView.setSocketReconnectInterval(60000);
|
|
});
|
|
Whisper.events.on('contactsync', function() {
|
|
if (appView.installView) {
|
|
appView.openInbox();
|
|
}
|
|
});
|
|
Whisper.events.on('contactsync:begin', function() {
|
|
if (appView.installView && appView.installView.showSync) {
|
|
appView.installView.showSync();
|
|
}
|
|
});
|
|
|
|
Whisper.Notifications.on('click', function(conversation) {
|
|
showWindow();
|
|
if (conversation) {
|
|
appView.openConversation(conversation);
|
|
} else {
|
|
appView.openInbox({
|
|
initialLoadComplete: initialLoadComplete
|
|
});
|
|
}
|
|
});
|
|
}
|
|
|
|
window.getSyncRequest = function() {
|
|
return new textsecure.SyncRequest(textsecure.messaging, messageReceiver);
|
|
};
|
|
|
|
Whisper.events.on('start-shutdown', function() {
|
|
if (messageReceiver) {
|
|
messageReceiver.close().then(function() {
|
|
messageReceiver = null;
|
|
Whisper.events.trigger('shutdown-complete');
|
|
});
|
|
} else {
|
|
Whisper.events.trigger('shutdown-complete');
|
|
}
|
|
});
|
|
|
|
function connect(firstRun) {
|
|
window.removeEventListener('online', connect);
|
|
|
|
if (!Whisper.Registration.isDone()) { return; }
|
|
if (Whisper.Import.isIncomplete()) { return; }
|
|
|
|
if (messageReceiver) { messageReceiver.close(); }
|
|
|
|
var USERNAME = storage.get('number_id');
|
|
var PASSWORD = storage.get('password');
|
|
var mySignalingKey = storage.get('signaling_key');
|
|
|
|
// initialize the socket and start listening for messages
|
|
messageReceiver = new textsecure.MessageReceiver(
|
|
SERVER_URL, USERNAME, PASSWORD, mySignalingKey
|
|
);
|
|
messageReceiver.addEventListener('message', onMessageReceived);
|
|
messageReceiver.addEventListener('receipt', onDeliveryReceipt);
|
|
messageReceiver.addEventListener('contact', onContactReceived);
|
|
messageReceiver.addEventListener('group', onGroupReceived);
|
|
messageReceiver.addEventListener('sent', onSentMessage);
|
|
messageReceiver.addEventListener('read', onReadReceipt);
|
|
messageReceiver.addEventListener('verified', onVerified);
|
|
messageReceiver.addEventListener('error', onError);
|
|
messageReceiver.addEventListener('empty', onEmpty);
|
|
messageReceiver.addEventListener('progress', onProgress);
|
|
|
|
window.textsecure.messaging = new textsecure.MessageSender(
|
|
SERVER_URL, USERNAME, PASSWORD
|
|
);
|
|
|
|
// Because v0.43.2 introduced a bug that lost contact details, v0.43.4 introduces
|
|
// a one-time contact sync to restore all lost contact/group information. We
|
|
// disable this checking if a user is first registering.
|
|
var key = 'chrome-contact-sync-v0.43.4';
|
|
if (!storage.get(key)) {
|
|
storage.put(key, true);
|
|
|
|
if (!firstRun && textsecure.storage.user.getDeviceId() != '1') {
|
|
window.getSyncRequest();
|
|
}
|
|
}
|
|
|
|
if (firstRun === true && textsecure.storage.user.getDeviceId() != '1') {
|
|
if (!storage.get('theme-setting') && textsecure.storage.get('userAgent') === 'OWI') {
|
|
storage.put('theme-setting', 'ios');
|
|
}
|
|
var syncRequest = new textsecure.SyncRequest(textsecure.messaging, messageReceiver);
|
|
Whisper.events.trigger('contactsync:begin');
|
|
syncRequest.addEventListener('success', function() {
|
|
console.log('sync successful');
|
|
storage.put('synced_at', Date.now());
|
|
Whisper.events.trigger('contactsync');
|
|
});
|
|
syncRequest.addEventListener('timeout', function() {
|
|
console.log('sync timed out');
|
|
Whisper.events.trigger('contactsync');
|
|
});
|
|
}
|
|
}
|
|
|
|
function onEmpty() {
|
|
initialLoadComplete = true;
|
|
|
|
var interval = setInterval(function() {
|
|
var view = window.owsDesktopApp.appView;
|
|
if (view) {
|
|
clearInterval(interval);
|
|
interval = null;
|
|
view.onEmpty();
|
|
}
|
|
}, 500);
|
|
}
|
|
function onProgress(ev) {
|
|
var count = ev.count;
|
|
|
|
var view = window.owsDesktopApp.appView;
|
|
if (view) {
|
|
view.onProgress(count);
|
|
}
|
|
}
|
|
|
|
function onContactReceived(ev) {
|
|
var details = ev.contactDetails;
|
|
|
|
var id = details.number;
|
|
var c = new Whisper.Conversation({
|
|
id: id
|
|
});
|
|
var error = c.validateNumber();
|
|
if (error) {
|
|
console.log('Invalid contact received', error && error.stack ? error.stack : error);
|
|
return;
|
|
}
|
|
|
|
return ConversationController.getOrCreateAndWait(id, 'private')
|
|
.then(function(conversation) {
|
|
return new Promise(function(resolve, reject) {
|
|
conversation.save({
|
|
name: details.name,
|
|
avatar: details.avatar,
|
|
color: details.color,
|
|
active_at: conversation.get('active_at') || Date.now(),
|
|
}).then(resolve, reject);
|
|
}).then(function() {
|
|
if (details.verified) {
|
|
var verified = details.verified;
|
|
var ev = new Event('verified');
|
|
ev.verified = {
|
|
state: verified.state,
|
|
destination: verified.destination,
|
|
identityKey: verified.identityKey.toArrayBuffer(),
|
|
};
|
|
ev.viaContactSync = true;
|
|
return onVerified(ev);
|
|
}
|
|
});
|
|
})
|
|
.then(ev.confirm)
|
|
.catch(function(error) {
|
|
console.log(
|
|
'onContactReceived error:',
|
|
error && error.stack ? error.stack : error
|
|
);
|
|
});
|
|
}
|
|
|
|
function onGroupReceived(ev) {
|
|
var details = ev.groupDetails;
|
|
var id = details.id;
|
|
|
|
return ConversationController.getOrCreateAndWait(id, 'group').then(function(conversation) {
|
|
var updates = {
|
|
name: details.name,
|
|
members: details.members,
|
|
avatar: details.avatar,
|
|
type: 'group',
|
|
};
|
|
if (details.active) {
|
|
updates.active_at = Date.now();
|
|
} else {
|
|
updates.left = true;
|
|
}
|
|
return new Promise(function(resolve, reject) {
|
|
conversation.save(updates).then(resolve, reject);
|
|
}).then(ev.confirm);
|
|
});
|
|
}
|
|
|
|
function onMessageReceived(ev) {
|
|
var data = ev.data;
|
|
var message = initIncomingMessage(data);
|
|
|
|
return isMessageDuplicate(message).then(function(isDuplicate) {
|
|
if (isDuplicate) {
|
|
console.log('Received duplicate message', message.idForLogging());
|
|
ev.confirm();
|
|
return;
|
|
}
|
|
|
|
var type, id;
|
|
if (data.message.group) {
|
|
type = 'group';
|
|
id = data.message.group.id;
|
|
} else {
|
|
type = 'private';
|
|
id = data.source;
|
|
}
|
|
|
|
return ConversationController.getOrCreateAndWait(id, type).then(function() {
|
|
return message.handleDataMessage(data.message, ev.confirm, {
|
|
initialLoadComplete: initialLoadComplete
|
|
});
|
|
});
|
|
});
|
|
}
|
|
|
|
function onSentMessage(ev) {
|
|
var now = new Date().getTime();
|
|
var data = ev.data;
|
|
|
|
var message = new Whisper.Message({
|
|
source : textsecure.storage.user.getNumber(),
|
|
sourceDevice : data.device,
|
|
sent_at : data.timestamp,
|
|
received_at : now,
|
|
conversationId : data.destination,
|
|
type : 'outgoing',
|
|
sent : true,
|
|
expirationStartTimestamp: data.expirationStartTimestamp,
|
|
});
|
|
|
|
return isMessageDuplicate(message).then(function(isDuplicate) {
|
|
if (isDuplicate) {
|
|
console.log('Received duplicate message', message.idForLogging());
|
|
ev.confirm();
|
|
return;
|
|
}
|
|
|
|
var type, id;
|
|
if (data.message.group) {
|
|
type = 'group';
|
|
id = data.message.group.id;
|
|
} else {
|
|
type = 'private';
|
|
id = data.destination;
|
|
}
|
|
|
|
return ConversationController.getOrCreateAndWait(id, type).then(function() {
|
|
return message.handleDataMessage(data.message, ev.confirm, {
|
|
initialLoadComplete: initialLoadComplete
|
|
});
|
|
});
|
|
});
|
|
}
|
|
|
|
function isMessageDuplicate(message) {
|
|
return new Promise(function(resolve) {
|
|
var fetcher = new Whisper.Message();
|
|
var options = {
|
|
index: {
|
|
name: 'unique',
|
|
value: [
|
|
message.get('source'),
|
|
message.get('sourceDevice'),
|
|
message.get('sent_at')
|
|
]
|
|
}
|
|
};
|
|
|
|
fetcher.fetch(options).always(function() {
|
|
if (fetcher.get('id')) {
|
|
return resolve(true);
|
|
}
|
|
|
|
return resolve(false);
|
|
});
|
|
}).catch(function(error) {
|
|
console.log('isMessageDuplicate error:', error && error.stack ? error.stack : error);
|
|
return false;
|
|
});
|
|
}
|
|
|
|
function initIncomingMessage(data) {
|
|
var message = new Whisper.Message({
|
|
source : data.source,
|
|
sourceDevice : data.sourceDevice,
|
|
sent_at : data.timestamp,
|
|
received_at : data.receivedAt || Date.now(),
|
|
conversationId : data.source,
|
|
type : 'incoming',
|
|
unread : 1
|
|
});
|
|
|
|
return message;
|
|
}
|
|
|
|
function onError(ev) {
|
|
var error = ev.error;
|
|
console.log(error);
|
|
console.log(error.stack);
|
|
|
|
if (error.name === 'HTTPError' && (error.code == 401 || error.code == 403)) {
|
|
Whisper.Registration.remove();
|
|
Whisper.events.trigger('unauthorized');
|
|
return;
|
|
}
|
|
|
|
if (error.name === 'HTTPError' && error.code == -1) {
|
|
// Failed to connect to server
|
|
if (navigator.onLine) {
|
|
console.log('retrying in 1 minute');
|
|
setTimeout(connect, 60000);
|
|
|
|
Whisper.events.trigger('reconnectTimer');
|
|
} else {
|
|
console.log('offline');
|
|
if (messageReceiver) { messageReceiver.close(); }
|
|
window.addEventListener('online', connect);
|
|
}
|
|
return;
|
|
}
|
|
|
|
if (ev.proto) {
|
|
if (error.name === 'MessageCounterError') {
|
|
if (ev.confirm) {
|
|
ev.confirm();
|
|
}
|
|
// Ignore this message. It is likely a duplicate delivery
|
|
// because the server lost our ack the first time.
|
|
return;
|
|
}
|
|
var envelope = ev.proto;
|
|
var message = initIncomingMessage(envelope);
|
|
|
|
return message.saveErrors(error).then(function() {
|
|
var id = message.get('conversationId');
|
|
return ConversationController.getOrCreateAndWait(id, 'private').then(function(conversation) {
|
|
conversation.set({
|
|
active_at: Date.now(),
|
|
unreadCount: conversation.get('unreadCount') + 1
|
|
});
|
|
|
|
var conversation_timestamp = conversation.get('timestamp');
|
|
var message_timestamp = message.get('timestamp');
|
|
if (!conversation_timestamp || message_timestamp > conversation_timestamp) {
|
|
conversation.set({ timestamp: message.get('sent_at') });
|
|
}
|
|
|
|
conversation.trigger('newmessage', message);
|
|
if (initialLoadComplete) {
|
|
conversation.notify(message);
|
|
}
|
|
|
|
if (ev.confirm) {
|
|
ev.confirm();
|
|
}
|
|
|
|
return new Promise(function(resolve, reject) {
|
|
conversation.save().then(resolve, reject);
|
|
});
|
|
});
|
|
});
|
|
}
|
|
|
|
throw error;
|
|
}
|
|
|
|
function onReadReceipt(ev) {
|
|
var read_at = ev.timestamp;
|
|
var timestamp = ev.read.timestamp;
|
|
var sender = ev.read.sender;
|
|
console.log('read receipt', sender, timestamp);
|
|
|
|
var receipt = Whisper.ReadReceipts.add({
|
|
sender : sender,
|
|
timestamp : timestamp,
|
|
read_at : read_at
|
|
});
|
|
|
|
receipt.on('remove', ev.confirm);
|
|
|
|
// Calling this directly so we can wait for completion
|
|
return Whisper.ReadReceipts.onReceipt(receipt);
|
|
}
|
|
|
|
function onVerified(ev) {
|
|
var number = ev.verified.destination;
|
|
var key = ev.verified.identityKey;
|
|
var state;
|
|
|
|
var c = new Whisper.Conversation({
|
|
id: number
|
|
});
|
|
var error = c.validateNumber();
|
|
if (error) {
|
|
console.log(
|
|
'Invalid verified sync received',
|
|
error && error.stack ? error.stack : error
|
|
);
|
|
return;
|
|
}
|
|
|
|
switch(ev.verified.state) {
|
|
case textsecure.protobuf.Verified.State.DEFAULT:
|
|
state = 'DEFAULT';
|
|
break;
|
|
case textsecure.protobuf.Verified.State.VERIFIED:
|
|
state = 'VERIFIED';
|
|
break;
|
|
case textsecure.protobuf.Verified.State.UNVERIFIED:
|
|
state = 'UNVERIFIED';
|
|
break;
|
|
}
|
|
|
|
console.log('got verified sync for', number, state,
|
|
ev.viaContactSync ? 'via contact sync' : '');
|
|
|
|
return ConversationController.getOrCreateAndWait(number, 'private').then(function(contact) {
|
|
var options = {
|
|
viaSyncMessage: true,
|
|
viaContactSync: ev.viaContactSync,
|
|
key: key
|
|
};
|
|
|
|
if (state === 'VERIFIED') {
|
|
return contact.setVerified(options).then(ev.confirm);
|
|
} else if (state === 'DEFAULT') {
|
|
return contact.setVerifiedDefault(options).then(ev.confirm);
|
|
} else {
|
|
return contact.setUnverified(options).then(ev.confirm);
|
|
}
|
|
});
|
|
}
|
|
|
|
function onDeliveryReceipt(ev) {
|
|
var pushMessage = ev.proto;
|
|
var timestamp = pushMessage.timestamp.toNumber();
|
|
console.log(
|
|
'delivery receipt from',
|
|
pushMessage.source + '.' + pushMessage.sourceDevice,
|
|
timestamp
|
|
);
|
|
|
|
var receipt = Whisper.DeliveryReceipts.add({
|
|
timestamp: timestamp,
|
|
source: pushMessage.source
|
|
});
|
|
|
|
receipt.on('remove', ev.confirm);
|
|
|
|
// Calling this directly so we can wait for completion
|
|
return Whisper.DeliveryReceipts.onReceipt(receipt);
|
|
}
|
|
})();
|