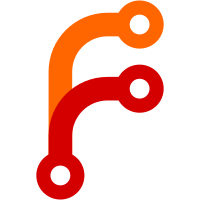
There are two parts to this change: 1. The computation of peaks is moved from `MessageAudio` to the `GlobalAudioContext` and thus we can limit the concurrency of the computations (`p-queue`!) and de-duplicate the computations as well 2. While the peaks are computed the component has to display spinning animation instead of empty waveform and unclickable UI.
39 lines
1.1 KiB
TypeScript
39 lines
1.1 KiB
TypeScript
// Copyright 2021 Signal Messenger, LLC
|
|
// SPDX-License-Identifier: AGPL-3.0-only
|
|
|
|
import { connect } from 'react-redux';
|
|
|
|
import { MessageAudio } from '../../components/conversation/MessageAudio';
|
|
import { ComputePeaksResult } from '../../components/GlobalAudioContext';
|
|
|
|
import { mapDispatchToProps } from '../actions';
|
|
import { StateType } from '../reducer';
|
|
import { LocalizerType } from '../../types/Util';
|
|
import { AttachmentType } from '../../types/Attachment';
|
|
|
|
export type Props = {
|
|
audio: HTMLAudioElement;
|
|
|
|
direction?: 'incoming' | 'outgoing';
|
|
id: string;
|
|
i18n: LocalizerType;
|
|
attachment: AttachmentType;
|
|
withContentAbove: boolean;
|
|
withContentBelow: boolean;
|
|
|
|
buttonRef: React.RefObject<HTMLButtonElement>;
|
|
|
|
computePeaks(url: string, barCount: number): Promise<ComputePeaksResult>;
|
|
kickOffAttachmentDownload(): void;
|
|
onCorrupted(): void;
|
|
};
|
|
|
|
const mapStateToProps = (state: StateType, props: Props) => {
|
|
return {
|
|
...props,
|
|
...state.audioPlayer,
|
|
};
|
|
};
|
|
|
|
const smart = connect(mapStateToProps, mapDispatchToProps);
|
|
export const SmartMessageAudio = smart(MessageAudio);
|