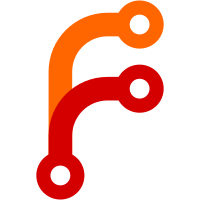
Biggest changes forced by this: alt tags for all images, resulting in new strings added to messages.json, and a new i18n paramter/prop added in a plot of places. Another change of note is that there are two new tslint.json files under ts/test and ts/styleguide to relax our rules a bit there. This required a change to our package.json script, as manually specifying the config file there made it ignore our tslint.json files in subdirectories
112 lines
2.4 KiB
TypeScript
112 lines
2.4 KiB
TypeScript
import React from 'react';
|
|
|
|
import moment from 'moment';
|
|
// tslint:disable-next-line:match-default-export-name
|
|
import formatFileSize from 'filesize';
|
|
|
|
import { Localizer } from '../../../types/Util';
|
|
|
|
interface Props {
|
|
// Required
|
|
i18n: Localizer;
|
|
timestamp: number;
|
|
|
|
// Optional
|
|
fileName?: string | null;
|
|
fileSize?: number;
|
|
onClick?: () => void;
|
|
shouldShowSeparator?: boolean;
|
|
}
|
|
|
|
const styles = {
|
|
container: {
|
|
width: '100%',
|
|
height: 72,
|
|
},
|
|
containerSeparator: {
|
|
borderBottomWidth: 1,
|
|
borderBottomColor: '#ccc',
|
|
borderBottomStyle: 'solid',
|
|
},
|
|
itemContainer: {
|
|
cursor: 'pointer',
|
|
display: 'flex',
|
|
flexDirection: 'row',
|
|
flexWrap: 'nowrap',
|
|
alignItems: 'center',
|
|
height: '100%',
|
|
} as React.CSSProperties,
|
|
itemMetadata: {
|
|
display: 'inline-flex',
|
|
flexDirection: 'column',
|
|
flexGrow: 1,
|
|
flexShrink: 0,
|
|
marginLeft: 8,
|
|
marginRight: 8,
|
|
} as React.CSSProperties,
|
|
itemDate: {
|
|
display: 'inline-block',
|
|
flexShrink: 0,
|
|
},
|
|
itemIcon: {
|
|
flexShrink: 0,
|
|
},
|
|
itemFileName: {
|
|
fontWeight: 'bold',
|
|
} as React.CSSProperties,
|
|
itemFileSize: {
|
|
display: 'inline-block',
|
|
marginTop: 8,
|
|
fontSize: '80%',
|
|
},
|
|
};
|
|
|
|
export class DocumentListItem extends React.Component<Props> {
|
|
public static defaultProps: Partial<Props> = {
|
|
shouldShowSeparator: true,
|
|
};
|
|
|
|
public render() {
|
|
const { shouldShowSeparator } = this.props;
|
|
|
|
return (
|
|
<div
|
|
style={{
|
|
...styles.container,
|
|
...(shouldShowSeparator ? styles.containerSeparator : {}),
|
|
}}
|
|
>
|
|
{this.renderContent()}
|
|
</div>
|
|
);
|
|
}
|
|
|
|
private renderContent() {
|
|
const { fileName, fileSize, timestamp, i18n } = this.props;
|
|
|
|
return (
|
|
<div
|
|
style={styles.itemContainer}
|
|
role="button"
|
|
onClick={this.props.onClick}
|
|
>
|
|
<img
|
|
alt={i18n('fileIconAlt')}
|
|
src="images/file.svg"
|
|
width="48"
|
|
height="48"
|
|
style={styles.itemIcon}
|
|
/>
|
|
<div style={styles.itemMetadata}>
|
|
<span style={styles.itemFileName}>{fileName}</span>
|
|
<span style={styles.itemFileSize}>
|
|
{typeof fileSize === 'number' ? formatFileSize(fileSize) : ''}
|
|
</span>
|
|
</div>
|
|
<div style={styles.itemDate}>
|
|
{moment(timestamp).format('ddd, MMM D, Y')}
|
|
</div>
|
|
</div>
|
|
);
|
|
}
|
|
}
|