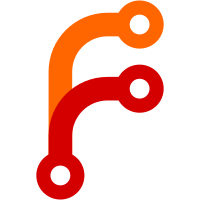
New experience in the Message Detail view when outgoing identity key errors happen, matching the Android View. 'View' button is only shown on outgoing key errors right now. When a contact with an outgoing identity key error is clicked, they are taken to a view like the popup that comes up on Android: an explanation of what happened and three options: 'Show Safety Number', 'Send Anyway', and 'Cancel' Contacts are now sorted alphabetically, with the set of contacts with errors coming before the rest. FREEBIE
51 lines
1.7 KiB
JavaScript
51 lines
1.7 KiB
JavaScript
/*
|
|
* vim: ts=4:sw=4:expandtab
|
|
*/
|
|
(function () {
|
|
'use strict';
|
|
window.Whisper = window.Whisper || {};
|
|
|
|
Whisper.IdentityKeySendErrorPanelView = Whisper.View.extend({
|
|
className: 'identity-key-send-error panel',
|
|
templateName: 'identity-key-send-error',
|
|
initialize: function(options) {
|
|
this.listenBack = options.listenBack;
|
|
this.resetPanel = options.resetPanel;
|
|
|
|
this.wasUnverified = this.model.isUnverified();
|
|
this.listenTo(this.model, 'change', this.render);
|
|
},
|
|
events: {
|
|
'click .show-safety-number': 'showSafetyNumber',
|
|
'click .send-anyway': 'sendAnyway',
|
|
'click .cancel': 'cancel'
|
|
},
|
|
showSafetyNumber: function() {
|
|
var view = new Whisper.KeyVerificationPanelView({
|
|
model: this.model
|
|
});
|
|
this.listenBack(view);
|
|
},
|
|
sendAnyway: function() {
|
|
this.resetPanel();
|
|
this.trigger('send-anyway');
|
|
},
|
|
cancel: function() {
|
|
this.resetPanel();
|
|
},
|
|
render_attributes: function() {
|
|
var send = i18n('sendAnyway');
|
|
if (this.wasUnverified && !this.model.isUnverified()) {
|
|
send = i18n('resend');
|
|
}
|
|
|
|
var errorExplanation = i18n('identityKeyErrorOnSend', this.model.getTitle(), this.model.getTitle());
|
|
return {
|
|
errorExplanation : errorExplanation,
|
|
showSafetyNumber : i18n('showSafetyNumber'),
|
|
sendAnyway : send,
|
|
cancel : i18n('cancel')
|
|
};
|
|
}
|
|
});
|
|
})();
|