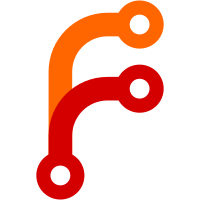
The Paper method was chosen for deprecation because it was more restrictive in that it has an isGliding check.
53 lines
2.4 KiB
Diff
53 lines
2.4 KiB
Diff
From 0000000000000000000000000000000000000000 Mon Sep 17 00:00:00 2001
|
|
From: Andrew Steinborn <git@steinborn.me>
|
|
Date: Mon, 9 Aug 2021 00:38:37 -0400
|
|
Subject: [PATCH] Optimize indirect passenger iteration
|
|
|
|
|
|
diff --git a/src/main/java/net/minecraft/world/entity/Entity.java b/src/main/java/net/minecraft/world/entity/Entity.java
|
|
index bbd071009d9ccf84f4c4ade296c98a8f4d6ac3ae..cfc9732378772084cd0badabaccd3de934b39596 100644
|
|
--- a/src/main/java/net/minecraft/world/entity/Entity.java
|
|
+++ b/src/main/java/net/minecraft/world/entity/Entity.java
|
|
@@ -3909,20 +3909,34 @@ public abstract class Entity implements Nameable, EntityAccess, CommandSource {
|
|
}
|
|
|
|
private Stream<Entity> getIndirectPassengersStream() {
|
|
+ if (this.passengers.isEmpty()) { return Stream.of(); } // Paper
|
|
return this.passengers.stream().flatMap(Entity::getSelfAndPassengers);
|
|
}
|
|
|
|
@Override
|
|
public Stream<Entity> getSelfAndPassengers() {
|
|
+ if (this.passengers.isEmpty()) { return Stream.of(this); } // Paper
|
|
return Stream.concat(Stream.of(this), this.getIndirectPassengersStream());
|
|
}
|
|
|
|
@Override
|
|
public Stream<Entity> getPassengersAndSelf() {
|
|
+ if (this.passengers.isEmpty()) { return Stream.of(this); } // Paper
|
|
return Stream.concat(this.passengers.stream().flatMap(Entity::getPassengersAndSelf), Stream.of(this));
|
|
}
|
|
|
|
public Iterable<Entity> getIndirectPassengers() {
|
|
+ // Paper start - rewrite this method
|
|
+ if (this.passengers.isEmpty()) { return ImmutableList.of(); }
|
|
+ ImmutableList.Builder<Entity> indirectPassengers = ImmutableList.builder();
|
|
+ for (Entity passenger : this.passengers) {
|
|
+ indirectPassengers.add(passenger);
|
|
+ indirectPassengers.addAll(passenger.getIndirectPassengers());
|
|
+ }
|
|
+ return indirectPassengers.build();
|
|
+ }
|
|
+ private Iterable<Entity> getIndirectPassengers_old() {
|
|
+ // Paper end
|
|
return () -> {
|
|
return this.getIndirectPassengersStream().iterator();
|
|
};
|
|
@@ -3939,6 +3953,7 @@ public abstract class Entity implements Nameable, EntityAccess, CommandSource {
|
|
// Paper end - rewrite chunk system
|
|
|
|
public boolean hasExactlyOnePlayerPassenger() {
|
|
+ if (this.passengers.isEmpty()) { return false; } // Paper
|
|
return this.getIndirectPassengersStream().filter((entity) -> {
|
|
return entity instanceof Player;
|
|
}).count() == 1L;
|