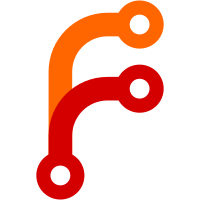
This does not change the overall license of the git-annex program, which was already AGPL due to a number of sources files being AGPL already. Legally speaking, I'm adding a new license under which these files are now available; I already released their current contents under the GPL license. Now they're dual licensed GPL and AGPL. However, I intend for all my future changes to these files to only be released under the AGPL license, and I won't be tracking the dual licensing status, so I'm simply changing the license statement to say it's AGPL. (In some cases, others wrote parts of the code of a file and released it under the GPL; but in all cases I have contributed a significant portion of the code in each file and it's that code that is getting the AGPL license; the GPL license of other contributors allows combining with AGPL code.)
66 lines
2 KiB
Haskell
66 lines
2 KiB
Haskell
{- P2P authtokens
|
|
-
|
|
- Copyright 2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module P2P.Auth where
|
|
|
|
import Annex.Common
|
|
import Creds
|
|
import P2P.Address
|
|
import Utility.AuthToken
|
|
import Utility.Tor
|
|
import Utility.Env
|
|
|
|
import qualified Data.Text as T
|
|
|
|
-- | Load authtokens that are accepted by this repository.
|
|
loadP2PAuthTokens :: Annex AllowedAuthTokens
|
|
loadP2PAuthTokens = allowedAuthTokens <$> loadP2PAuthTokens'
|
|
|
|
loadP2PAuthTokens' :: Annex [AuthToken]
|
|
loadP2PAuthTokens' = mapMaybe toAuthToken
|
|
. map T.pack
|
|
. lines
|
|
. fromMaybe []
|
|
<$> readCreds p2pAuthCredsFile
|
|
|
|
-- | Stores an AuthToken, making it be accepted by this repository.
|
|
storeP2PAuthToken :: AuthToken -> Annex ()
|
|
storeP2PAuthToken t = do
|
|
ts <- loadP2PAuthTokens'
|
|
unless (t `elem` ts) $ do
|
|
let d = unlines $ map (T.unpack . fromAuthToken) (t:ts)
|
|
writeCreds d p2pAuthCredsFile
|
|
|
|
p2pAuthCredsFile :: FilePath
|
|
p2pAuthCredsFile = "p2pauth"
|
|
|
|
-- | Loads the AuthToken to use when connecting with a given P2P address.
|
|
--
|
|
-- It's loaded from the first line of the creds file, but
|
|
-- GIT_ANNEX_P2P_AUTHTOKEN overrides.
|
|
loadP2PRemoteAuthToken :: P2PAddress -> Annex (Maybe AuthToken)
|
|
loadP2PRemoteAuthToken addr = maybe Nothing mk <$> getM id
|
|
[ liftIO $ getEnv "GIT_ANNEX_P2P_AUTHTOKEN"
|
|
, readCreds (addressCredsFile addr)
|
|
]
|
|
where
|
|
mk = toAuthToken . T.pack . takeWhile (/= '\n')
|
|
|
|
p2pAuthTokenEnv :: String
|
|
p2pAuthTokenEnv = "GIT_ANNEX_P2P_AUTHTOKEN"
|
|
|
|
-- | Stores the AuthToken to use when connecting with a given P2P address.
|
|
storeP2PRemoteAuthToken :: P2PAddress -> AuthToken -> Annex ()
|
|
storeP2PRemoteAuthToken addr t = writeCreds
|
|
(T.unpack $ fromAuthToken t)
|
|
(addressCredsFile addr)
|
|
|
|
addressCredsFile :: P2PAddress -> FilePath
|
|
-- We can omit the port and just use the onion address for the creds file,
|
|
-- because any given tor hidden service runs on a single port and has a
|
|
-- unique onion address.
|
|
addressCredsFile (TorAnnex (OnionAddress onionaddr) _port) = onionaddr
|