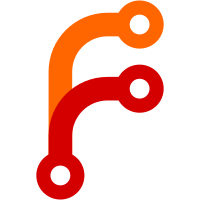
This does not change the overall license of the git-annex program, which was already AGPL due to a number of sources files being AGPL already. Legally speaking, I'm adding a new license under which these files are now available; I already released their current contents under the GPL license. Now they're dual licensed GPL and AGPL. However, I intend for all my future changes to these files to only be released under the AGPL license, and I won't be tracking the dual licensing status, so I'm simply changing the license statement to say it's AGPL. (In some cases, others wrote parts of the code of a file and released it under the GPL; but in all cases I have contributed a significant portion of the code in each file and it's that code that is getting the AGPL license; the GPL license of other contributors allows combining with AGPL code.)
72 lines
2.1 KiB
Haskell
72 lines
2.1 KiB
Haskell
{- GIT_SSH and GIT_SSH_COMMAND support
|
|
-
|
|
- Copyright 2017 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Git.Ssh (module Git.Ssh, module Utility.SshHost) where
|
|
|
|
import Common
|
|
import Utility.Env
|
|
import Utility.SshHost
|
|
|
|
import Data.Char
|
|
|
|
gitSshEnv :: String
|
|
gitSshEnv = "GIT_SSH"
|
|
|
|
gitSshCommandEnv :: String
|
|
gitSshCommandEnv = "GIT_SSH_COMMAND"
|
|
|
|
gitSshEnvSet :: IO Bool
|
|
gitSshEnvSet = anyM (isJust <$$> getEnv) [gitSshEnv, gitSshCommandEnv]
|
|
|
|
type SshPort = Integer
|
|
|
|
-- Command to run on the remote host. It is run by the shell
|
|
-- there, so any necessary shell escaping of parameters in it should
|
|
-- already be done.
|
|
type SshCommand = String
|
|
|
|
-- | Checks for GIT_SSH and GIT_SSH_COMMAND and if set, returns
|
|
-- a command and parameters to run to ssh.
|
|
gitSsh :: SshHost -> Maybe SshPort -> SshCommand -> IO (Maybe (FilePath, [CommandParam]))
|
|
gitSsh host mp cmd = gitSsh' host mp cmd []
|
|
|
|
gitSsh' :: SshHost -> Maybe SshPort -> SshCommand -> [CommandParam] -> IO (Maybe (FilePath, [CommandParam]))
|
|
gitSsh' host mp cmd extrasshparams = do
|
|
gsc <- getEnv gitSshCommandEnv
|
|
case gsc of
|
|
Just c
|
|
-- git only runs the command with the shell
|
|
-- when it contains spaces; otherwise it's
|
|
-- treated the same as GIT_SSH
|
|
| any isSpace c -> ret "sh"
|
|
[ Param "-c"
|
|
, Param (shellcmd c sshps)
|
|
]
|
|
| otherwise -> ret c sshps
|
|
Nothing -> do
|
|
gs <- getEnv gitSshEnv
|
|
case gs of
|
|
Just c -> ret c sshps
|
|
Nothing -> return Nothing
|
|
where
|
|
ret c l = return $ Just (c, l)
|
|
|
|
-- Git passes exactly these parameters to the ssh command.
|
|
gitps = map Param $ case mp of
|
|
Nothing -> [fromSshHost host, cmd]
|
|
Just p -> [fromSshHost host, "-p", show p, cmd]
|
|
|
|
-- Passing any extra parameters to the ssh command may
|
|
-- break some commands.
|
|
sshps = extrasshparams ++ gitps
|
|
|
|
-- The shell command to run with sh -c is constructed
|
|
-- this way, rather than using "$@" because there could be some
|
|
-- unwanted parameters passed to the command, and this way they
|
|
-- are ignored. For example, when Utility.Rsync.rsyncShell is
|
|
-- used, rsync adds some parameters after the command.
|
|
shellcmd c ps = c ++ " " ++ unwords (map shellEscape (toCommand ps))
|