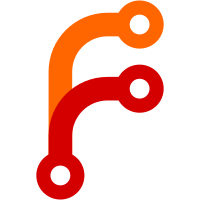
Works around this bug in unix-compat: https://github.com/jacobstanley/unix-compat/issues/56 getFileStatus and other FilePath using functions in unix-compat do not do UNC conversion on Windows. Made Utility.RawFilePath use convertToWindowsNativeNamespace to do the necessary conversion on windows to support long filenames. Audited all imports of System.PosixCompat.Files to make sure that no functions that operate on FilePath were imported from it. Instead, use the equvilants from Utility.RawFilePath. In particular the re-export of that module in Common had to be removed, which led to lots of other changes throughout the code. The changes to Build.Configure, Build.DesktopFile, and Build.TestConfig make Utility.Directory not be needed to build setup. And so let it use Utility.RawFilePath, which depends on unix, which cannot be in setup-depends. Sponsored-by: Dartmouth College's Datalad project
98 lines
3 KiB
Haskell
98 lines
3 KiB
Haskell
{- P2P protocol addresses
|
|
-
|
|
- Copyright 2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module P2P.Address where
|
|
|
|
import qualified Annex
|
|
import Annex.Common
|
|
import Git
|
|
import Git.Types
|
|
import Creds
|
|
import Utility.AuthToken
|
|
import Utility.Tor
|
|
import qualified Utility.RawFilePath as R
|
|
|
|
import qualified Data.Text as T
|
|
import System.PosixCompat.Files (fileOwner, fileGroup)
|
|
|
|
-- | A P2P address, without an AuthToken.
|
|
--
|
|
-- This is enough information to connect to the peer,
|
|
-- but not enough to authenticate with it.
|
|
data P2PAddress = TorAnnex OnionAddress OnionPort
|
|
deriving (Eq, Show)
|
|
|
|
-- | A P2P address, with an AuthToken.
|
|
--
|
|
-- This is enough information to connect to the peer, and authenticate with
|
|
-- it.
|
|
data P2PAddressAuth = P2PAddressAuth P2PAddress AuthToken
|
|
deriving (Eq, Show)
|
|
|
|
class FormatP2PAddress a where
|
|
formatP2PAddress :: a -> String
|
|
unformatP2PAddress :: String -> Maybe a
|
|
|
|
instance FormatP2PAddress P2PAddress where
|
|
formatP2PAddress (TorAnnex (OnionAddress onionaddr) onionport) =
|
|
torAnnexScheme ++ ":" ++ onionaddr ++ ":" ++ show onionport
|
|
unformatP2PAddress s
|
|
| (torAnnexScheme ++ ":") `isPrefixOf` s = do
|
|
let s' = dropWhile (== ':') $ dropWhile (/= ':') s
|
|
let (onionaddr, ps) = separate (== ':') s'
|
|
onionport <- readish ps
|
|
return (TorAnnex (OnionAddress onionaddr) onionport)
|
|
| otherwise = Nothing
|
|
|
|
torAnnexScheme :: String
|
|
torAnnexScheme = "tor-annex:"
|
|
|
|
instance FormatP2PAddress P2PAddressAuth where
|
|
formatP2PAddress (P2PAddressAuth addr authtoken) =
|
|
formatP2PAddress addr ++ ":" ++ T.unpack (fromAuthToken authtoken)
|
|
unformatP2PAddress s = do
|
|
let (ra, rs) = separate (== ':') (reverse s)
|
|
addr <- unformatP2PAddress (reverse rs)
|
|
authtoken <- toAuthToken (T.pack $ reverse ra)
|
|
return (P2PAddressAuth addr authtoken)
|
|
|
|
repoP2PAddress :: Repo -> Maybe P2PAddress
|
|
repoP2PAddress (Repo { location = Url url }) = unformatP2PAddress (show url)
|
|
repoP2PAddress _ = Nothing
|
|
|
|
-- | Load known P2P addresses for this repository.
|
|
loadP2PAddresses :: Annex [P2PAddress]
|
|
loadP2PAddresses = mapMaybe unformatP2PAddress . maybe [] lines
|
|
<$> readCreds p2pAddressCredsFile
|
|
|
|
-- | Store a new P2P address for this repository.
|
|
storeP2PAddress :: P2PAddress -> Annex ()
|
|
storeP2PAddress addr = do
|
|
addrs <- loadP2PAddresses
|
|
unless (addr `elem` addrs) $ do
|
|
let s = unlines $ map formatP2PAddress (addr:addrs)
|
|
let tmpnam = p2pAddressCredsFile ++ ".new"
|
|
writeCreds s tmpnam
|
|
tmpf <- credsFile tmpnam
|
|
destf <- credsFile p2pAddressCredsFile
|
|
-- This may be run by root, so make the creds file
|
|
-- and directory have the same owner and group as
|
|
-- the git repository directory has.
|
|
st <- liftIO . R.getFileStatus . toRawFilePath
|
|
=<< Annex.fromRepo repoLocation
|
|
let fixowner f = R.setOwnerAndGroup (toRawFilePath f) (fileOwner st) (fileGroup st)
|
|
liftIO $ do
|
|
fixowner tmpf
|
|
fixowner (takeDirectory tmpf)
|
|
fixowner (takeDirectory (takeDirectory tmpf))
|
|
renameFile tmpf destf
|
|
|
|
p2pAddressCredsFile :: FilePath
|
|
p2pAddressCredsFile = "p2paddrs"
|
|
|
|
torAppName :: AppName
|
|
torAppName = "tor-annex"
|