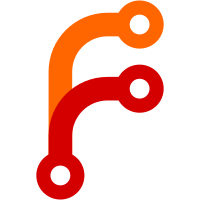
WIP: This is mostly complete, but there is a problem: createDirectoryUnder throws an error when annex.dbdir is set to outside the git repo. annex.dbdir is a workaround for filesystems where sqlite does not work, due to eg, the filesystem not properly supporting locking. It's intended to be set before initializing the repository. Changing it in an existing repository can be done, but would be the same as making a new repository and moving all the annexed objects into it. While the databases get recreated from the git-annex branch in that situation, any information that is in the databases but not stored in the branch gets lost. It may be that no information ever gets stored in the databases that cannot be reconstructed from the branch, but I have not verified that. Sponsored-by: Dartmouth College's Datalad project
53 lines
1.3 KiB
Haskell
53 lines
1.3 KiB
Haskell
{- git-annex upgrade log
|
|
-
|
|
- This file is stored locally in .git/annex/, not in the git-annex branch.
|
|
-
|
|
- The format: "version timestamp"
|
|
-
|
|
- Copyright 2022 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.Upgrade (
|
|
writeUpgradeLog,
|
|
readUpgradeLog,
|
|
timeOfUpgrade,
|
|
) where
|
|
|
|
import Annex.Common
|
|
import Utility.TimeStamp
|
|
import Logs.File
|
|
import Types.RepoVersion
|
|
|
|
import Data.Time.Clock.POSIX
|
|
|
|
writeUpgradeLog :: RepoVersion -> POSIXTime-> Annex ()
|
|
writeUpgradeLog v t = do
|
|
logf <- fromRepo gitAnnexUpgradeLog
|
|
lckf <- fromRepo gitAnnexUpgradeLock
|
|
appendLogFile logf lckf $ encodeBL $
|
|
show (fromRepoVersion v) ++ " " ++ show t
|
|
|
|
readUpgradeLog :: Annex [(RepoVersion, POSIXTime)]
|
|
readUpgradeLog = do
|
|
logfile <- fromRawFilePath <$> fromRepo gitAnnexUpgradeLog
|
|
ifM (liftIO $ doesFileExist logfile)
|
|
( mapMaybe parse . lines
|
|
<$> liftIO (readFileStrict logfile)
|
|
, return []
|
|
)
|
|
where
|
|
parse line = case (readish sint, parsePOSIXTime ts) of
|
|
(Just v, Just t) -> Just (RepoVersion v, t)
|
|
_ -> Nothing
|
|
where
|
|
(sint, ts) = separate (== ' ') line
|
|
|
|
timeOfUpgrade :: RepoVersion -> Annex (Maybe POSIXTime)
|
|
timeOfUpgrade want = do
|
|
l <- readUpgradeLog
|
|
return $ case filter (\(v, _) -> v == want) l of
|
|
[] -> Nothing
|
|
l' -> Just (minimum (map snd l'))
|
|
|