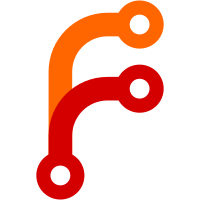
Noticed that Semigroup instance of Map is not suitable to use for MapLog. For example, it behaved like this: ghci> parseTrustLog "foo 1 timestamp=10\nfoo 2 timestamp=11" <> parseTrustLog "foo X timestamp=12" fromList [(UUID "foo",LogEntry {changed = VectorClock 11s, value = SemiTrusted})] Which was wrong, it lost the newer DeadTrusted value. Luckily, nothing used that Semigroup when operating on a MapLog. And this provides a safe instance. Sponsored-by: Graham Spencer on Patreon
49 lines
1.3 KiB
Haskell
49 lines
1.3 KiB
Haskell
{- Remote state logs.
|
|
-
|
|
- Copyright 2014, 2019 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Logs.RemoteState (
|
|
getRemoteState,
|
|
setRemoteState,
|
|
) where
|
|
|
|
import Annex.Common
|
|
import Types.RemoteState
|
|
import Logs
|
|
import Logs.UUIDBased
|
|
import Logs.MapLog
|
|
import qualified Annex.Branch
|
|
import qualified Annex
|
|
|
|
import qualified Data.Map as M
|
|
import qualified Data.ByteString.Lazy as L
|
|
import qualified Data.Attoparsec.ByteString.Lazy as A
|
|
import Data.ByteString.Builder
|
|
|
|
type RemoteState = String
|
|
|
|
setRemoteState :: RemoteStateHandle -> Key -> RemoteState -> Annex ()
|
|
setRemoteState (RemoteStateHandle u) k s = do
|
|
c <- currentVectorClock
|
|
config <- Annex.getGitConfig
|
|
Annex.Branch.change
|
|
(Annex.Branch.RegardingUUID [u])
|
|
(remoteStateLogFile config k)
|
|
(buildRemoteState . changeLog c u s . parseRemoteState)
|
|
|
|
buildRemoteState :: Log RemoteState -> Builder
|
|
buildRemoteState = buildLogNew (byteString . encodeBS)
|
|
|
|
getRemoteState :: RemoteStateHandle -> Key -> Annex (Maybe RemoteState)
|
|
getRemoteState (RemoteStateHandle u) k = do
|
|
config <- Annex.getGitConfig
|
|
extract . fromMapLog . parseRemoteState
|
|
<$> Annex.Branch.get (remoteStateLogFile config k)
|
|
where
|
|
extract m = value <$> M.lookup u m
|
|
|
|
parseRemoteState :: L.ByteString -> Log RemoteState
|
|
parseRemoteState = parseLogNew (decodeBS <$> A.takeByteString)
|