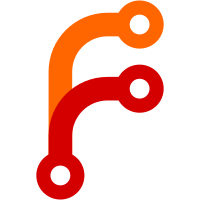
When --batch is used with matching options like --in, --metadata, etc, only operate on the provided files when they match those options. Otherwise, a blank line is output in the batch protocol. Affected commands: find, add, whereis, drop, copy, move, get In the case of find, the documentation for --batch already said it honored the matching options. The docs for the rest didn't, but it makes sense to have them honor them. While this is a behavior change, why specify the matching options with --batch if you didn't want them to apply? Note that the batch output for all of the affected commands could already output a blank line in other cases, so batch users should already be prepared to deal with it. git-annex metadata didn't seem worth making support the matching options, since all it does is output metadata or set metadata, the use cases for using it in combination with the martching options seem small. Made it refuse to run when they're combined, leaving open the possibility for later support if a use case develops. This commit was sponsored by Brett Eisenberg on Patreon.
91 lines
2.8 KiB
Haskell
91 lines
2.8 KiB
Haskell
{- git-annex batch commands
|
|
-
|
|
- Copyright 2015 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module CmdLine.Batch where
|
|
|
|
import Annex.Common
|
|
import Types.Command
|
|
import CmdLine.Action
|
|
import CmdLine.GitAnnex.Options
|
|
import Options.Applicative
|
|
import Limit
|
|
import Types.FileMatcher
|
|
|
|
data BatchMode = Batch | NoBatch
|
|
|
|
parseBatchOption :: Parser BatchMode
|
|
parseBatchOption = flag NoBatch Batch
|
|
( long "batch"
|
|
<> help "enable batch mode"
|
|
)
|
|
|
|
-- A batchable command can run in batch mode, or not.
|
|
-- In batch mode, one line at a time is read, parsed, and a reply output to
|
|
-- stdout. In non batch mode, the command's parameters are parsed and
|
|
-- a reply output for each.
|
|
batchable :: (opts -> String -> Annex Bool) -> Parser opts -> CmdParamsDesc -> CommandParser
|
|
batchable handler parser paramdesc = batchseeker <$> batchparser
|
|
where
|
|
batchparser = (,,)
|
|
<$> parser
|
|
<*> parseBatchOption
|
|
<*> cmdParams paramdesc
|
|
|
|
batchseeker (opts, NoBatch, params) = mapM_ (go NoBatch opts) params
|
|
batchseeker (opts, Batch, _) = batchInput Right (go Batch opts)
|
|
|
|
go batchmode opts p =
|
|
unlessM (handler opts p) $
|
|
batchBadInput batchmode
|
|
|
|
-- bad input is indicated by an empty line in batch mode. In non batch
|
|
-- mode, exit on bad input.
|
|
batchBadInput :: BatchMode -> Annex ()
|
|
batchBadInput NoBatch = liftIO exitFailure
|
|
batchBadInput Batch = liftIO $ putStrLn ""
|
|
|
|
-- Reads lines of batch mode input and passes to the action to handle.
|
|
batchInput :: (String -> Either String a) -> (a -> Annex ()) -> Annex ()
|
|
batchInput parser a = go =<< batchLines
|
|
where
|
|
go [] = return ()
|
|
go (l:rest) = do
|
|
either parseerr a (parser l)
|
|
go rest
|
|
parseerr s = giveup $ "Batch input parse failure: " ++ s
|
|
|
|
batchLines :: Annex [String]
|
|
batchLines = liftIO $ lines <$> getContents
|
|
|
|
-- Runs a CommandStart in batch mode.
|
|
--
|
|
-- The batch mode user expects to read a line of output, and it's up to the
|
|
-- CommandStart to generate that output as it succeeds or fails to do its
|
|
-- job. However, if it stops without doing anything, it won't generate
|
|
-- any output, so in that case, batchBadInput is used to provide the caller
|
|
-- with an empty line.
|
|
batchCommandAction :: CommandStart -> Annex ()
|
|
batchCommandAction a = maybe (batchBadInput Batch) (const noop)
|
|
=<< callCommandAction' a
|
|
|
|
-- Reads lines of batch input and passes the filepaths to a CommandStart
|
|
-- to handle them.
|
|
--
|
|
-- File matching options are not checked.
|
|
allBatchFiles :: (FilePath -> CommandStart) -> Annex ()
|
|
allBatchFiles a = batchInput Right $ batchCommandAction . a
|
|
|
|
-- Like allBatchFiles, but checks the file matching options
|
|
-- and skips non-matching files.
|
|
batchFilesMatching :: (FilePath -> CommandStart) -> Annex ()
|
|
batchFilesMatching a = do
|
|
matcher <- getMatcher
|
|
allBatchFiles $ \f ->
|
|
ifM (matcher $ MatchingFile $ FileInfo f f)
|
|
( a f
|
|
, return Nothing
|
|
)
|