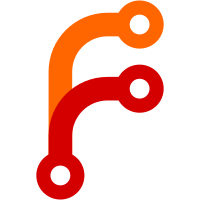
Straightforward, except for the issue of how to reverse LockAdjustment. With --unlock, a commit that modifies/adds unlocked files gets reverse adjusted to use locked files. That's fairly reasonable, I think. But reversing --lock by unlocking all modified files feels wrong. Maybe that's just because repositories typically seem to still have mostly locked files in them (unless one is in an adjusted unlocked branch of course!) It may be that eventually how to reverse both will need to be configurable, I don't know.
54 lines
1.7 KiB
Haskell
54 lines
1.7 KiB
Haskell
{- adjusted branch types
|
|
-
|
|
- Copyright 2016-2018 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.AdjustedBranch where
|
|
|
|
data Adjustment
|
|
= LinkAdjustment LinkAdjustment
|
|
| PresenceAdjustment PresenceAdjustment (Maybe LinkAdjustment)
|
|
deriving (Show, Eq)
|
|
|
|
-- Doesn't make sense to combine unlock with fix.
|
|
data LinkAdjustment
|
|
= UnlockAdjustment
|
|
| LockAdjustment
|
|
| FixAdjustment
|
|
| UnFixAdjustment
|
|
deriving (Show, Eq)
|
|
|
|
data PresenceAdjustment
|
|
= HideMissingAdjustment
|
|
| ShowMissingAdjustment
|
|
deriving (Show, Eq)
|
|
|
|
-- Adjustments have to be able to be reversed, so that commits made to the
|
|
-- adjusted branch can be reversed to the commit that would have been made
|
|
-- without the adjustment and applied to the original branch.
|
|
class ReversableAdjustment t where
|
|
reverseAdjustment :: t -> t
|
|
|
|
instance ReversableAdjustment Adjustment where
|
|
reverseAdjustment (LinkAdjustment l) =
|
|
LinkAdjustment (reverseAdjustment l)
|
|
reverseAdjustment (PresenceAdjustment p ml) =
|
|
PresenceAdjustment (reverseAdjustment p) (fmap reverseAdjustment ml)
|
|
|
|
instance ReversableAdjustment LinkAdjustment where
|
|
reverseAdjustment UnlockAdjustment = LockAdjustment
|
|
-- Keep the file locked intentionally when reversing LockAdjustment.
|
|
reverseAdjustment LockAdjustment = LockAdjustment
|
|
reverseAdjustment FixAdjustment = UnFixAdjustment
|
|
reverseAdjustment UnFixAdjustment = FixAdjustment
|
|
|
|
instance ReversableAdjustment PresenceAdjustment where
|
|
reverseAdjustment HideMissingAdjustment = ShowMissingAdjustment
|
|
reverseAdjustment ShowMissingAdjustment = HideMissingAdjustment
|
|
|
|
adjustmentHidesFiles :: Adjustment -> Bool
|
|
adjustmentHidesFiles (PresenceAdjustment HideMissingAdjustment _) = True
|
|
adjustmentHidesFiles _ = False
|
|
|