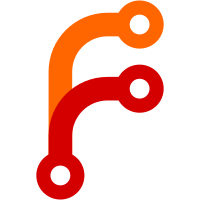
Removed old extensible-exceptions, only needed for very old ghc. Made webdav use Utility.Exception, to work after some changes in DAV's exception handling. Removed Annex.Exception. Mostly this was trivial, but note that tryAnnex is replaced with tryNonAsync and catchAnnex replaced with catchNonAsync. In theory that could be a behavior change, since the former caught all exceptions, and the latter don't catch async exceptions. However, in practice, nothing in the Annex monad uses async exceptions. Grepping for throwTo and killThread only find stuff in the assistant, which does not seem related. Command.Add.undo is changed to accept a SomeException, and things that use it for rollback now catch non-async exceptions, rather than only IOExceptions.
34 lines
899 B
Haskell
34 lines
899 B
Haskell
{- parallel processing via threads
|
|
-
|
|
- Copyright 2012 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- License: BSD-2-clause
|
|
-}
|
|
|
|
module Utility.Parallel where
|
|
|
|
import Common
|
|
|
|
import Control.Concurrent
|
|
|
|
{- Runs an action in parallel with a set of values, in a set of threads.
|
|
- In order for the actions to truely run in parallel, requires GHC's
|
|
- threaded runtime,
|
|
-
|
|
- Returns the values partitioned into ones with which the action succeeded,
|
|
- and ones with which it failed. -}
|
|
inParallel :: (v -> IO Bool) -> [v] -> IO ([v], [v])
|
|
inParallel a l = do
|
|
mvars <- mapM thread l
|
|
statuses <- mapM takeMVar mvars
|
|
return $ reduce $ partition snd $ zip l statuses
|
|
where
|
|
reduce (x,y) = (map fst x, map fst y)
|
|
thread v = do
|
|
mvar <- newEmptyMVar
|
|
_ <- forkIO $ do
|
|
r <- try (a v) :: IO (Either SomeException Bool)
|
|
case r of
|
|
Left _ -> putMVar mvar False
|
|
Right b -> putMVar mvar b
|
|
return mvar
|