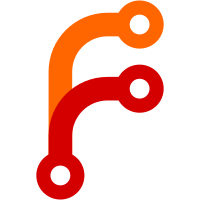
Ignore annex.numcopies set to 0 in gitattributes or git config, or by git-annex numcopies or by --numcopies, since that configuration would make git-annex easily lose data. Same for mincopies. This is a continuation of the work to make data only be able to be lost when --force is used. It earlier led to the --trust option being disabled, and similar reasoning applies here. Most numcopies configs had docs that strongly discouraged setting it to 0 anyway. And I can't imagine a use case for setting to 0. Not that there might not be one, but it's just so far from the intended use case of git-annex, of managing and storing your data, that it does not seem like it makes sense to cater to such a hypothetical use case, where any git-annex drop can lose your data at any time. Using a smart constructor makes sure every place avoids 0. Note that this does mean that NumCopies is for the configured desired values, and not the actual existing number of copies, which of course can be 0. The name configuredNumCopies is used to make that clear. Sponsored-by: Brock Spratlen on Patreon
66 lines
1.9 KiB
Haskell
66 lines
1.9 KiB
Haskell
{- git-annex numcopies log
|
|
-
|
|
- Copyright 2014-2021 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# OPTIONS_GHC -fno-warn-orphans #-}
|
|
|
|
module Logs.NumCopies (
|
|
setGlobalNumCopies,
|
|
setGlobalMinCopies,
|
|
getGlobalNumCopies,
|
|
getGlobalMinCopies,
|
|
globalNumCopiesLoad,
|
|
globalMinCopiesLoad,
|
|
) where
|
|
|
|
import Annex.Common
|
|
import qualified Annex
|
|
import qualified Annex.Branch
|
|
import Types.NumCopies
|
|
import Logs
|
|
import Logs.SingleValue
|
|
|
|
instance SingleValueSerializable NumCopies where
|
|
serialize = encodeBS . show . fromNumCopies
|
|
deserialize = configuredNumCopies <$$> readish . decodeBS
|
|
|
|
instance SingleValueSerializable MinCopies where
|
|
serialize = encodeBS . show . fromMinCopies
|
|
deserialize = configuredMinCopies <$$> readish . decodeBS
|
|
|
|
setGlobalNumCopies :: NumCopies -> Annex ()
|
|
setGlobalNumCopies new = do
|
|
curr <- getGlobalNumCopies
|
|
when (curr /= Just new) $
|
|
setLog (Annex.Branch.RegardingUUID []) numcopiesLog new
|
|
|
|
setGlobalMinCopies :: MinCopies -> Annex ()
|
|
setGlobalMinCopies new = do
|
|
curr <- getGlobalMinCopies
|
|
when (curr /= Just new) $
|
|
setLog (Annex.Branch.RegardingUUID []) mincopiesLog new
|
|
|
|
{- Value configured in the numcopies log. Cached for speed. -}
|
|
getGlobalNumCopies :: Annex (Maybe NumCopies)
|
|
getGlobalNumCopies = maybe globalNumCopiesLoad (return . Just)
|
|
=<< Annex.getState Annex.globalnumcopies
|
|
|
|
{- Value configured in the mincopies log. Cached for speed. -}
|
|
getGlobalMinCopies :: Annex (Maybe MinCopies)
|
|
getGlobalMinCopies = maybe globalMinCopiesLoad (return . Just)
|
|
=<< Annex.getState Annex.globalmincopies
|
|
|
|
globalNumCopiesLoad :: Annex (Maybe NumCopies)
|
|
globalNumCopiesLoad = do
|
|
v <- getLog numcopiesLog
|
|
Annex.changeState $ \s -> s { Annex.globalnumcopies = v }
|
|
return v
|
|
|
|
globalMinCopiesLoad :: Annex (Maybe MinCopies)
|
|
globalMinCopiesLoad = do
|
|
v <- getLog mincopiesLog
|
|
Annex.changeState $ \s -> s { Annex.globalmincopies = v }
|
|
return v
|