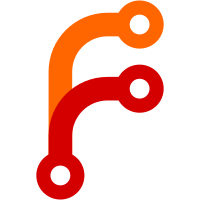
Fix a crash opening sqlite databases when run in a non-unicode locale, with a remote that uses a non-unicode filepath. In that situation converting to Text fails. The fix needs git-annex to be built with persistent-sqlite 2.13.3. Building against older versions still works, but that version is used when building with stack. Database.RawFilePath is a lot of code copied from persistent-sqlite and lightly modified, since only 1 function in persistent-sqlite was made to support RawFilePath. This is a bit of a pain, and I hope that persistent-sqlite will eventually switch to using OsPath, allowing this module to be removed from git-annex. Sponsored-by: k0ld on Patreon
64 lines
2 KiB
Haskell
64 lines
2 KiB
Haskell
{- Persistent sqlite database initialization
|
|
-
|
|
- Copyright 2015-2023 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
{-# LANGUAGE OverloadedStrings, CPP #-}
|
|
|
|
module Database.Init where
|
|
|
|
import Annex.Common
|
|
import Annex.Perms
|
|
import Utility.FileMode
|
|
import qualified Utility.RawFilePath as R
|
|
#if MIN_VERSION_persistent_sqlite(2,13,3)
|
|
import Database.RawFilePath
|
|
#endif
|
|
|
|
import Database.Persist.Sqlite
|
|
import Lens.Micro
|
|
import qualified Data.Text as T
|
|
import qualified System.FilePath.ByteString as P
|
|
|
|
{- Ensures that the database is freshly initialized. Deletes any
|
|
- existing database. Pass the migration action for the database.
|
|
-
|
|
- The permissions of the database are set based on the
|
|
- core.sharedRepository setting. Setting these permissions on the main db
|
|
- file causes Sqlite to always use the same permissions for additional
|
|
- files it writes later on
|
|
-}
|
|
initDb :: P.RawFilePath -> SqlPersistM () -> Annex ()
|
|
initDb db migration = do
|
|
let dbdir = P.takeDirectory db
|
|
let tmpdbdir = dbdir <> ".tmp"
|
|
let tmpdb = tmpdbdir P.</> "db"
|
|
let tmpdb' = T.pack (fromRawFilePath tmpdb)
|
|
createAnnexDirectory tmpdbdir
|
|
#if MIN_VERSION_persistent_sqlite(2,13,3)
|
|
liftIO $ runSqliteInfo' tmpdb (enableWAL tmpdb') migration
|
|
#else
|
|
liftIO $ runSqliteInfo (enableWAL tmpdb') migration
|
|
#endif
|
|
setAnnexDirPerm tmpdbdir
|
|
-- Work around sqlite bug that prevents it from honoring
|
|
-- less restrictive umasks.
|
|
liftIO $ R.setFileMode tmpdb =<< defaultFileMode
|
|
setAnnexFilePerm tmpdb
|
|
liftIO $ do
|
|
void $ tryIO $ removeDirectoryRecursive (fromRawFilePath dbdir)
|
|
R.rename tmpdbdir dbdir
|
|
|
|
{- Make sure that the database uses WAL mode, to prevent readers
|
|
- from blocking writers, and prevent a writer from blocking readers.
|
|
-
|
|
- This is the default in recent persistent-sqlite versions, but
|
|
- force it on just in case.
|
|
-
|
|
- Note that once WAL mode is enabled, it will persist whenever the
|
|
- database is opened. -}
|
|
enableWAL :: T.Text -> SqliteConnectionInfo
|
|
enableWAL db = over walEnabled (const True) $
|
|
mkSqliteConnectionInfo db
|