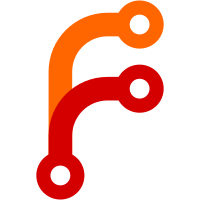
The journal read optimisation inaeca7c220
later got fixed ineedd73b84
to stage and commit any files that were left in the journal by a previous git-annex run. That's necessary for the optimisation to work correctly. But it also meant that alwayscommit=false started committing the previous git-annex processes journalled changes, which defeated the purpose of the config setting entirely. So, disable the optimisation when alwayscommit=false, leaving the files in the journal and not committing them. See my comments on the bug report for why this seemed the best approach. Also fixes a problem when annex.merge-annex-branches=false and there are changes in the journal. That config indirectly prevents committing the journal. (Which seems a bit odd given its name, but it always has..) So, when there were changes in the journal, perhaps left there due to alwayscommit=false being set before, the optimisation would prevent git-annex from reading the journal files, and it would operate with out of date information.
82 lines
2.7 KiB
Haskell
82 lines
2.7 KiB
Haskell
{- git-annex branch state management
|
|
-
|
|
- Runtime state about the git-annex branch.
|
|
-
|
|
- Copyright 2011-2020 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Annex.BranchState where
|
|
|
|
import Annex.Common
|
|
import Types.BranchState
|
|
import qualified Annex
|
|
|
|
getState :: Annex BranchState
|
|
getState = Annex.getState Annex.branchstate
|
|
|
|
changeState :: (BranchState -> BranchState) -> Annex ()
|
|
changeState changer = Annex.changeState $ \s ->
|
|
s { Annex.branchstate = changer (Annex.branchstate s) }
|
|
|
|
{- Runs an action to check that the index file exists, if it's not been
|
|
- checked before in this run of git-annex. -}
|
|
checkIndexOnce :: Annex () -> Annex ()
|
|
checkIndexOnce a = unlessM (indexChecked <$> getState) $ do
|
|
a
|
|
changeState $ \s -> s { indexChecked = True }
|
|
|
|
{- Runs an action to update the branch, if it's not been updated before
|
|
- in this run of git-annex.
|
|
-
|
|
- The action should return True if anything that was in the journal
|
|
- before got staged (or if the journal was empty). That lets an opmisation
|
|
- be done: The journal then does not need to be checked going forward,
|
|
- until new information gets written to it.
|
|
-}
|
|
runUpdateOnce :: Annex Bool -> Annex BranchState
|
|
runUpdateOnce a = do
|
|
st <- getState
|
|
if branchUpdated st
|
|
then return st
|
|
else do
|
|
journalstaged <- a
|
|
let stf = \st' -> st'
|
|
{ branchUpdated = True
|
|
, journalIgnorable = journalstaged
|
|
&& not (journalNeverIgnorable st')
|
|
}
|
|
changeState stf
|
|
return (stf st)
|
|
|
|
{- Avoids updating the branch. A useful optimisation when the branch
|
|
- is known to have not changed, or git-annex won't be relying on info
|
|
- from it. -}
|
|
disableUpdate :: Annex ()
|
|
disableUpdate = changeState $ \s -> s { branchUpdated = True }
|
|
|
|
{- Called when a change is made to the journal. -}
|
|
journalChanged :: Annex ()
|
|
journalChanged = do
|
|
-- Optimisation: Typically journalIgnorable will already be True
|
|
-- (when one thing gets journalled, often other things do to),
|
|
-- so avoid an unnecessary write to the MVar that changeState
|
|
-- would do.
|
|
--
|
|
-- This assumes that another thread is not changing journalIgnorable
|
|
-- at the same time, but since runUpdateOnce is the only
|
|
-- thing that changes it, and it only runs once, that
|
|
-- should not happen.
|
|
st <- getState
|
|
when (journalIgnorable st) $
|
|
changeState $ \st' -> st' { journalIgnorable = False }
|
|
|
|
{- When git-annex is somehow interactive, eg in --batch mode,
|
|
- and needs to always notice changes made to the journal by other
|
|
- processes, this disables optimisations that avoid normally reading the
|
|
- journal.
|
|
-}
|
|
enableInteractiveJournalAccess :: Annex ()
|
|
enableInteractiveJournalAccess = changeState $
|
|
\s -> s { journalNeverIgnorable = True }
|