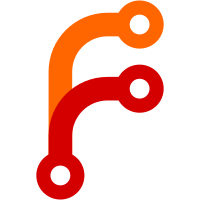
* rmurl: When youtube-dl was used for an url, it no longer needs to be prefixed with "yt:" in order to be removed. * rmurl: If an url is both used by the web and also claimed by another special remote, fix a bug that caused the url to to not be removed. The youtube-dl change is a consequence of how the bug fix is implemented. But I also think it's the right thing to do. Consider that, before, git-annex addurl $url followed by git-annex rmurl $url would not remove the url in the case where youtube-dl was used. That was surprising behavior. In the unlikely case where a special remote claims an url, and it's been added using OtherDownloader, but it was also added already as a web url, it seems better for rmurl to remove both than to arbitrarily remove only one. And in the case the bug report was filed for, when an url was added as a web url, but a special remote now claims it, that should not prevent rmurl removing the web url. Calling setUrlMissing lets other callers of it behave differently. Probably the calls to it in eg, Remote.External and Remote.BitTorrent are fine, since they don't mangle the url and just remove what was provided, and the OtherDownloader form of a bittorrent url, respectively. I suspect unregisterurl needs to have a similar change made to rmurl, for similar reasons.
60 lines
1.7 KiB
Haskell
60 lines
1.7 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2013-2021 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.RmUrl where
|
|
|
|
import Command
|
|
import Logs.Web
|
|
|
|
cmd :: Command
|
|
cmd = notBareRepo $
|
|
command "rmurl" SectionCommon
|
|
"record file is not available at url"
|
|
(paramRepeating (paramPair paramFile paramUrl))
|
|
(seek <$$> optParser)
|
|
|
|
data RmUrlOptions = RmUrlOptions
|
|
{ rmThese :: CmdParams
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser RmUrlOptions
|
|
optParser desc = RmUrlOptions
|
|
<$> cmdParams desc
|
|
<*> parseBatchOption
|
|
|
|
seek :: RmUrlOptions -> CommandSeek
|
|
seek o = case batchOption o of
|
|
Batch fmt -> batchInput fmt batchParser (batchCommandAction . start)
|
|
NoBatch -> withPairs (commandAction . start) (rmThese o)
|
|
|
|
-- Split on the last space, since a FilePath can contain whitespace,
|
|
-- but a url should not.
|
|
batchParser :: String -> Annex (Either String (FilePath, URLString))
|
|
batchParser s = case separate (== ' ') (reverse s) of
|
|
(ru, rf)
|
|
| null ru || null rf -> return $ Left "Expected: \"file url\""
|
|
| otherwise -> do
|
|
let f = reverse rf
|
|
f' <- liftIO $ fromRawFilePath
|
|
<$> relPathCwdToFile (toRawFilePath f)
|
|
return $ Right (f', reverse ru)
|
|
|
|
start :: (SeekInput, (FilePath, URLString)) -> CommandStart
|
|
start (si, (file, url)) = flip whenAnnexed file' $ \_ key -> do
|
|
let ai = mkActionItem (key, AssociatedFile (Just file'))
|
|
starting "rmurl" ai si $
|
|
next $ cleanup url key
|
|
where
|
|
file' = toRawFilePath file
|
|
|
|
cleanup :: String -> Key -> CommandCleanup
|
|
cleanup url key = do
|
|
-- Remove the url, no matter what downloader.
|
|
forM_ [minBound..maxBound] $ \dl ->
|
|
setUrlMissing key (setDownloader url dl)
|
|
return True
|