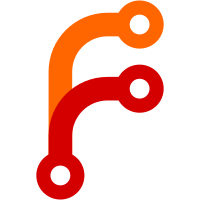
This avoids all the complication about redundant work discussed in the previous try at fixing this. At the expense of needing each command that could have the problem to be patched to simply wrap the action in onlyActionOn once the key is known. But there do not seem to be many such commands. onlyActionOn' should not be used with a CommandStart (or CommandPerform), although the types do allow it. onlyActionOn handles running the whole CommandStart chain. I couldn't immediately see a way to avoid mistken use of onlyActionOn'. This commit was supported by the NSF-funded DataLad project.
68 lines
1.9 KiB
Haskell
68 lines
1.9 KiB
Haskell
{- git-annex transfer types
|
|
-
|
|
- Copyright 2012 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.Transfer where
|
|
|
|
import Types
|
|
import Utility.PID
|
|
import Utility.QuickCheck
|
|
|
|
import Data.Time.Clock.POSIX
|
|
import Control.Concurrent
|
|
import Control.Applicative
|
|
import Prelude
|
|
|
|
{- Enough information to uniquely identify a transfer. -}
|
|
data Transfer = Transfer
|
|
{ transferDirection :: Direction
|
|
, transferUUID :: UUID
|
|
, transferKey :: Key
|
|
}
|
|
deriving (Eq, Ord, Read, Show)
|
|
|
|
{- Information about a Transfer, stored in the transfer information file.
|
|
-
|
|
- Note that the associatedFile may not correspond to a file in the local
|
|
- git repository. It's some file, possibly relative to some directory,
|
|
- of some repository, that was acted on to initiate the transfer.
|
|
-}
|
|
data TransferInfo = TransferInfo
|
|
{ startedTime :: Maybe POSIXTime
|
|
, transferPid :: Maybe PID
|
|
, transferTid :: Maybe ThreadId
|
|
, transferRemote :: Maybe Remote
|
|
, bytesComplete :: Maybe Integer
|
|
, associatedFile :: AssociatedFile
|
|
, transferPaused :: Bool
|
|
}
|
|
deriving (Show, Eq, Ord)
|
|
|
|
stubTransferInfo :: TransferInfo
|
|
stubTransferInfo = TransferInfo Nothing Nothing Nothing Nothing Nothing (AssociatedFile Nothing) False
|
|
|
|
data Direction = Upload | Download
|
|
deriving (Eq, Ord, Show, Read)
|
|
|
|
formatDirection :: Direction -> String
|
|
formatDirection Upload = "upload"
|
|
formatDirection Download = "download"
|
|
|
|
parseDirection :: String -> Maybe Direction
|
|
parseDirection "upload" = Just Upload
|
|
parseDirection "download" = Just Download
|
|
parseDirection _ = Nothing
|
|
|
|
instance Arbitrary TransferInfo where
|
|
arbitrary = TransferInfo
|
|
<$> arbitrary
|
|
<*> arbitrary
|
|
<*> pure Nothing -- cannot generate a ThreadID
|
|
<*> pure Nothing -- remote not needed
|
|
<*> arbitrary
|
|
-- associated file cannot be empty (but can be Nothing)
|
|
<*> (AssociatedFile <$> arbitrary `suchThat` (/= Just ""))
|
|
<*> arbitrary
|