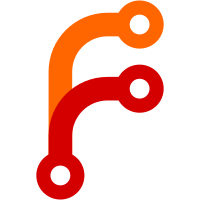
Added annex.bwlimit and remote.name.annex-bwlimit config that works for git remotes and many but not all special remotes. This nearly works, at least for a git remote on the same disk. With it set to 100kb/1s, the meter displays an actual bandwidth of 128 kb/s, with occasional spikes to 160 kb/s. So it needs to delay just a bit longer... I'm unsure why. However, at the beginning a lot of data flows before it determines the right bandwidth limit. A granularity of less than 1s would probably improve that. And, I don't know yet if it makes sense to have it be 100ks/1s rather than 100kb/s. Is there a situation where the user would want a larger granularity? Does granulatity need to be configurable at all? I only used that format for the config really in order to reuse an existing parser. This can't support for external special remotes, or for ones that themselves shell out to an external command. (Well, it could, but it would involve pausing and resuming the child process tree, which seems very hard to implement and very strange besides.) There could also be some built-in special remotes that it still doesn't work for, due to them not having a progress meter whose displays blocks the bandwidth using thread. But I don't think there are actually any that run a separate thread for downloads than the thread that displays the progress meter. Sponsored-by: Graham Spencer on Patreon
50 lines
1.3 KiB
Haskell
50 lines
1.3 KiB
Haskell
{- types for stall detection and banwdith rates
|
|
-
|
|
- Copyright 2020-2021 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.StallDetection where
|
|
|
|
import Utility.DataUnits
|
|
import Utility.HumanTime
|
|
import Utility.Misc
|
|
import Git.Config
|
|
|
|
data StallDetection
|
|
= StallDetection BwRate
|
|
-- ^ Unless the given number of bytes have been sent over the given
|
|
-- amount of time, there's a stall.
|
|
| ProbeStallDetection
|
|
-- ^ Used when unsure how frequently transfer progress is updated,
|
|
-- or how fast data can be sent.
|
|
| StallDetectionDisabled
|
|
deriving (Show)
|
|
|
|
data BwRate = BwRate ByteSize Duration
|
|
deriving (Show)
|
|
|
|
-- Parse eg, "0KiB/60s"
|
|
--
|
|
-- Also, it can be set to "true" (or other git config equivilants)
|
|
-- to enable ProbeStallDetection.
|
|
-- And "false" (and other git config equivilants) explicitly
|
|
-- disable stall detection.
|
|
parseStallDetection :: String -> Either String StallDetection
|
|
parseStallDetection s = case isTrueFalse s of
|
|
Nothing -> do
|
|
v <- parseBwRate s
|
|
Right (StallDetection v)
|
|
Just True -> Right ProbeStallDetection
|
|
Just False -> Right StallDetectionDisabled
|
|
|
|
parseBwRate :: String -> Either String BwRate
|
|
parseBwRate s = do
|
|
let (bs, ds) = separate (== '/') s
|
|
b <- maybe
|
|
(Left $ "Unable to parse bandwidth amount " ++ bs)
|
|
Right
|
|
(readSize dataUnits bs)
|
|
d <- parseDuration ds
|
|
Right (BwRate b d)
|