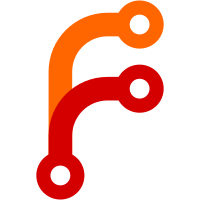
p2p and multicast creds are not cached the same way that s3 and webdav creds are. The difference is that p2p and multicast obtain the creds themselves, as part of a process like pairing. So they're storing the only extant copy of the creds. In s3 and webdav etc the creds are provided by the cloud storage provider. This is a fine difference, but I do think it's a reasonable difference. If the user wants to prevent s3 and webdav etc creds from being stored unencrypted on disk, they won't feel the same about p2p auth tokens used for tor, or a multicast encryption key, or for that matter their local ssh private key. This commit was sponsored by Fernando Jimenez on Patreon.
66 lines
2 KiB
Haskell
66 lines
2 KiB
Haskell
{- P2P authtokens
|
|
-
|
|
- Copyright 2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module P2P.Auth where
|
|
|
|
import Annex.Common
|
|
import Creds
|
|
import P2P.Address
|
|
import Utility.AuthToken
|
|
import Utility.Tor
|
|
import Utility.Env
|
|
|
|
import qualified Data.Text as T
|
|
|
|
-- | Load authtokens that are accepted by this repository.
|
|
loadP2PAuthTokens :: Annex AllowedAuthTokens
|
|
loadP2PAuthTokens = allowedAuthTokens <$> loadP2PAuthTokens'
|
|
|
|
loadP2PAuthTokens' :: Annex [AuthToken]
|
|
loadP2PAuthTokens' = mapMaybe toAuthToken
|
|
. map T.pack
|
|
. lines
|
|
. fromMaybe []
|
|
<$> readCreds p2pAuthCredsFile
|
|
|
|
-- | Stores an AuthToken, making it be accepted by this repository.
|
|
storeP2PAuthToken :: AuthToken -> Annex ()
|
|
storeP2PAuthToken t = do
|
|
ts <- loadP2PAuthTokens'
|
|
unless (t `elem` ts) $ do
|
|
let d = unlines $ map (T.unpack . fromAuthToken) (t:ts)
|
|
writeCreds d p2pAuthCredsFile
|
|
|
|
p2pAuthCredsFile :: FilePath
|
|
p2pAuthCredsFile = "p2pauth"
|
|
|
|
-- | Loads the AuthToken to use when connecting with a given P2P address.
|
|
--
|
|
-- It's loaded from the first line of the creds file, but
|
|
-- GIT_ANNEX_P2P_AUTHTOKEN overrides.
|
|
loadP2PRemoteAuthToken :: P2PAddress -> Annex (Maybe AuthToken)
|
|
loadP2PRemoteAuthToken addr = maybe Nothing mk <$> getM id
|
|
[ liftIO $ getEnv "GIT_ANNEX_P2P_AUTHTOKEN"
|
|
, readCreds (addressCredsFile addr)
|
|
]
|
|
where
|
|
mk = toAuthToken . T.pack . takeWhile (/= '\n')
|
|
|
|
p2pAuthTokenEnv :: String
|
|
p2pAuthTokenEnv = "GIT_ANNEX_P2P_AUTHTOKEN"
|
|
|
|
-- | Stores the AuthToken to use when connecting with a given P2P address.
|
|
storeP2PRemoteAuthToken :: P2PAddress -> AuthToken -> Annex ()
|
|
storeP2PRemoteAuthToken addr t = writeCreds
|
|
(T.unpack $ fromAuthToken t)
|
|
(addressCredsFile addr)
|
|
|
|
addressCredsFile :: P2PAddress -> FilePath
|
|
-- We can omit the port and just use the onion address for the creds file,
|
|
-- because any given tor hidden service runs on a single port and has a
|
|
-- unique onion address.
|
|
addressCredsFile (TorAnnex (OnionAddress onionaddr) _port) = onionaddr
|