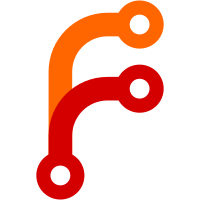
There is no suitable git hook to run code when pulling changes that might need to be merged into the git-annex branch. The post-merge hook is only run when changes are merged into HEAD, and it's possible, and indeed likely that many pulls will only have changes in git-annex, but not in HEAD, and not trigger it. So, git-annex will have to take care to update the branch before reading from it, to make sure it has merged in current info from remotes. Happily, this can be done quite inexpensively, just a git-show-ref to list branches, and a minimalized git-log to see if there are unmerged changes on the branches. To further speed up, it will be done only once per git-annex run, max.
99 lines
2.2 KiB
Haskell
99 lines
2.2 KiB
Haskell
{- git-annex monad
|
||
-
|
||
- Copyright 2010 Joey Hess <joey@kitenet.net>
|
||
-
|
||
- Licensed under the GNU GPL version 3 or higher.
|
||
-}
|
||
|
||
module Annex (
|
||
Annex,
|
||
AnnexState(..),
|
||
new,
|
||
run,
|
||
eval,
|
||
getState,
|
||
changeState,
|
||
gitRepo
|
||
) where
|
||
|
||
import Control.Monad.State
|
||
|
||
import qualified GitRepo as Git
|
||
import GitQueue
|
||
import Types.Backend
|
||
import Types.Remote
|
||
import Types.Crypto
|
||
import TrustLevel
|
||
import Types.UUID
|
||
|
||
-- git-annex's monad
|
||
type Annex = StateT AnnexState IO
|
||
|
||
-- internal state storage
|
||
data AnnexState = AnnexState
|
||
{ repo :: Git.Repo
|
||
, backends :: [Backend Annex]
|
||
, supportedBackends :: [Backend Annex]
|
||
, remotes :: [Remote Annex]
|
||
, repoqueue :: Queue
|
||
, quiet :: Bool
|
||
, force :: Bool
|
||
, fast :: Bool
|
||
, updated :: Bool
|
||
, forcebackend :: Maybe String
|
||
, forcenumcopies :: Maybe Int
|
||
, defaultkey :: Maybe String
|
||
, toremote :: Maybe String
|
||
, fromremote :: Maybe String
|
||
, exclude :: [String]
|
||
, forcetrust :: [(UUID, TrustLevel)]
|
||
, cipher :: Maybe Cipher
|
||
}
|
||
|
||
newState :: [Backend Annex] -> Git.Repo -> AnnexState
|
||
newState allbackends gitrepo = AnnexState
|
||
{ repo = gitrepo
|
||
, backends = []
|
||
, remotes = []
|
||
, supportedBackends = allbackends
|
||
, repoqueue = empty
|
||
, quiet = False
|
||
, force = False
|
||
, fast = False
|
||
, updated = False
|
||
, forcebackend = Nothing
|
||
, forcenumcopies = Nothing
|
||
, defaultkey = Nothing
|
||
, toremote = Nothing
|
||
, fromremote = Nothing
|
||
, exclude = []
|
||
, forcetrust = []
|
||
, cipher = Nothing
|
||
}
|
||
|
||
{- Create and returns an Annex state object for the specified git repo. -}
|
||
new :: Git.Repo -> [Backend Annex] -> IO AnnexState
|
||
new gitrepo allbackends =
|
||
newState allbackends `liftM` (liftIO . Git.configRead) gitrepo
|
||
|
||
{- performs an action in the Annex monad -}
|
||
run :: AnnexState -> Annex a -> IO (a, AnnexState)
|
||
run = flip runStateT
|
||
eval :: AnnexState -> Annex a -> IO a
|
||
eval = flip evalStateT
|
||
|
||
{- Gets a value from the internal state, selected by the passed value
|
||
- constructor. -}
|
||
getState :: (AnnexState -> a) -> Annex a
|
||
getState = gets
|
||
|
||
{- Applies a state mutation function to change the internal state.
|
||
-
|
||
- Example: changeState $ \s -> s { quiet = True }
|
||
-}
|
||
changeState :: (AnnexState -> AnnexState) -> Annex ()
|
||
changeState = modify
|
||
|
||
{- Returns the git repository being acted on -}
|
||
gitRepo :: Annex Git.Repo
|
||
gitRepo = getState repo
|