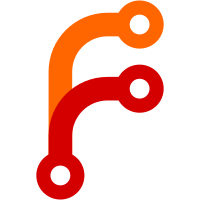
Added --json-error-messages option, which includes error messages in the json output, rather than outputting them to stderr. The actual rediretion of errors is not implemented yet, this is only the docs and option plumbing. This commit was supported by the NSF-funded DataLad project.
59 lines
1.4 KiB
Haskell
59 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010,2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.DropKey where
|
|
|
|
import Command
|
|
import qualified Annex
|
|
import Logs.Location
|
|
import Annex.Content
|
|
|
|
cmd :: Command
|
|
cmd = noCommit $ withGlobalOptions [jsonOptions] $
|
|
command "dropkey" SectionPlumbing
|
|
"drops annexed content for specified keys"
|
|
(paramRepeating paramKey)
|
|
(seek <$$> optParser)
|
|
|
|
data DropKeyOptions = DropKeyOptions
|
|
{ toDrop :: [String]
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser DropKeyOptions
|
|
optParser desc = DropKeyOptions
|
|
<$> cmdParams desc
|
|
<*> parseBatchOption
|
|
|
|
seek :: DropKeyOptions -> CommandSeek
|
|
seek o = do
|
|
unlessM (Annex.getState Annex.force) $
|
|
giveup "dropkey can cause data loss; use --force if you're sure you want to do this"
|
|
withKeys start (toDrop o)
|
|
case batchOption o of
|
|
Batch -> batchInput parsekey $ batchCommandAction . start
|
|
NoBatch -> noop
|
|
where
|
|
parsekey = maybe (Left "bad key") Right . file2key
|
|
|
|
start :: Key -> CommandStart
|
|
start key = do
|
|
showStartKey "dropkey" key (mkActionItem key)
|
|
next $ perform key
|
|
|
|
perform :: Key -> CommandPerform
|
|
perform key = ifM (inAnnex key)
|
|
( lockContentForRemoval key $ \contentlock -> do
|
|
removeAnnex contentlock
|
|
next $ cleanup key
|
|
, next $ return True
|
|
)
|
|
|
|
cleanup :: Key -> CommandCleanup
|
|
cleanup key = do
|
|
logStatus key InfoMissing
|
|
return True
|