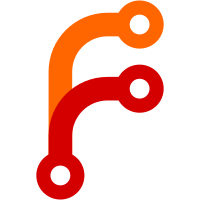
Luckily, this did not affect any git-annex log files, since they all include the trailing 's' for backwards compatability reasons. But, if I later want to drop that, this is the first commit where git-annex can be trusted to parse that right. The misparse caused it to be off by up to 10 seconds.
43 lines
1.1 KiB
Haskell
43 lines
1.1 KiB
Haskell
{- timestamp parsing and formatting
|
|
-
|
|
- Copyright 2015-2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- License: BSD-2-clause
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module Utility.TimeStamp where
|
|
|
|
import Utility.PartialPrelude
|
|
import Utility.Misc
|
|
|
|
import Data.Time.Clock.POSIX
|
|
import Data.Time
|
|
import Data.Ratio
|
|
#if ! MIN_VERSION_time(1,5,0)
|
|
import System.Locale
|
|
#endif
|
|
|
|
{- Parses how POSIXTime shows itself: "1431286201.113452s"
|
|
- Also handles the format with no fractional seconds. -}
|
|
parsePOSIXTime :: String -> Maybe POSIXTime
|
|
parsePOSIXTime = uncurry parsePOSIXTime' . separate (== '.')
|
|
|
|
{- Parses the integral and decimal part of a POSIXTime -}
|
|
parsePOSIXTime' :: String -> String -> Maybe POSIXTime
|
|
parsePOSIXTime' sn sd = do
|
|
n <- readi sn
|
|
let sd' = takeWhile (/= 's') sd
|
|
if null sd'
|
|
then return (fromIntegral n)
|
|
else do
|
|
d <- readi sd'
|
|
let r = d % (10 ^ (length sd'))
|
|
return (fromIntegral n + fromRational r)
|
|
where
|
|
readi :: String -> Maybe Integer
|
|
readi = readish
|
|
|
|
formatPOSIXTime :: String -> POSIXTime -> String
|
|
formatPOSIXTime fmt t = formatTime defaultTimeLocale fmt (posixSecondsToUTCTime t)
|