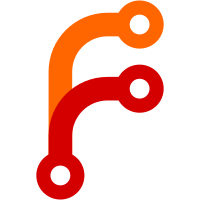
When in a subdir, both the normal filepath, and the filepath relative to the top of the git repo are needed for matching. The former for key lookup, and the latter for include/exclude to match against. Previously, key lookup didn't work in this situation.
33 lines
1 KiB
Haskell
33 lines
1 KiB
Haskell
{- git-annex control over whether content is wanted
|
|
-
|
|
- Copyright 2012 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Annex.Wanted where
|
|
|
|
import Common.Annex
|
|
import Logs.PreferredContent
|
|
import Annex.UUID
|
|
import Types.Remote
|
|
|
|
import qualified Data.Set as S
|
|
|
|
{- Check if a file is preferred content for the local repository. -}
|
|
wantGet :: AssociatedFile -> Annex Bool
|
|
wantGet Nothing = return True
|
|
wantGet (Just file) = isPreferredContent Nothing S.empty file
|
|
|
|
{- Check if a file is preferred content for a remote. -}
|
|
wantSend :: AssociatedFile -> UUID -> Annex Bool
|
|
wantSend Nothing _ = return True
|
|
wantSend (Just file) to = isPreferredContent (Just to) S.empty file
|
|
|
|
{- Check if a file can be dropped, maybe from a remote.
|
|
- Don't drop files that are preferred content. -}
|
|
wantDrop :: Maybe UUID -> AssociatedFile -> Annex Bool
|
|
wantDrop _ Nothing = return True
|
|
wantDrop from (Just file) = do
|
|
u <- maybe getUUID (return . id) from
|
|
not <$> isPreferredContent (Just u) (S.singleton u) file
|