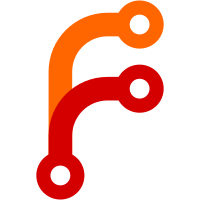
In git, a Ref can be a Sha, or a Branch, or a Tag. I added type aliases for those. Note that this does not prevent mixing up of eg, refs and branches at the type level. Since git really doesn't care, except rare cases like git update-ref, or git tag -d, that seems ok for now. There's also a tree-ish, but let's just use Ref for it. A given Sha or Ref may or may not be a tree-ish, depending on the object type, so there seems no point in trying to represent it at the type level.
46 lines
982 B
Haskell
46 lines
982 B
Haskell
{- git-union-merge program
|
|
-
|
|
- Copyright 2011 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
import System.Environment
|
|
|
|
import Common
|
|
import qualified Git.UnionMerge
|
|
import qualified Git
|
|
|
|
header :: String
|
|
header = "Usage: git-union-merge ref ref newref"
|
|
|
|
usage :: IO a
|
|
usage = error $ "bad parameters\n\n" ++ header
|
|
|
|
tmpIndex :: Git.Repo -> FilePath
|
|
tmpIndex g = Git.gitDir g </> "index.git-union-merge"
|
|
|
|
setup :: Git.Repo -> IO ()
|
|
setup = cleanup -- idempotency
|
|
|
|
cleanup :: Git.Repo -> IO ()
|
|
cleanup g = do
|
|
e' <- doesFileExist (tmpIndex g)
|
|
when e' $ removeFile (tmpIndex g)
|
|
|
|
parseArgs :: IO [String]
|
|
parseArgs = do
|
|
args <- getArgs
|
|
if length args /= 3
|
|
then usage
|
|
else return args
|
|
|
|
main :: IO ()
|
|
main = do
|
|
[aref, bref, newref] <- map Git.Ref <$> parseArgs
|
|
g <- Git.configRead =<< Git.repoFromCwd
|
|
_ <- Git.useIndex (tmpIndex g)
|
|
setup g
|
|
Git.UnionMerge.merge aref bref g
|
|
Git.commit "union merge" newref [aref, bref] g
|
|
cleanup g
|