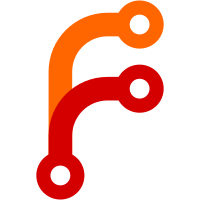
This allows the Backend type to not depend on the Annex type, and so the Annex type can later be moved out of TypeInternals.
59 lines
1.4 KiB
Haskell
59 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.Unannex where
|
|
|
|
import Control.Monad.State (liftIO)
|
|
import System.Directory
|
|
|
|
import Command
|
|
import qualified Annex
|
|
import Utility
|
|
import qualified Backend
|
|
import LocationLog
|
|
import Types
|
|
import Content
|
|
import qualified GitRepo as Git
|
|
import Messages
|
|
|
|
command :: [Command]
|
|
command = [Command "unannex" paramPath seek "undo accidential add command"]
|
|
|
|
seek :: [CommandSeek]
|
|
seek = [withFilesInGit start]
|
|
|
|
{- The unannex subcommand undoes an add. -}
|
|
start :: CommandStartString
|
|
start file = isAnnexed file $ \(key, backend) -> do
|
|
ishere <- inAnnex key
|
|
if ishere
|
|
then do
|
|
showStart "unannex" file
|
|
return $ Just $ perform file key backend
|
|
else return Nothing
|
|
|
|
perform :: FilePath -> Key -> Backend Annex -> CommandPerform
|
|
perform file key backend = do
|
|
-- force backend to always remove
|
|
ok <- Backend.removeKey backend key (Just 0)
|
|
if ok
|
|
then return $ Just $ cleanup file key
|
|
else return Nothing
|
|
|
|
cleanup :: FilePath -> Key -> CommandCleanup
|
|
cleanup file key = do
|
|
g <- Annex.gitRepo
|
|
|
|
liftIO $ removeFile file
|
|
liftIO $ Git.run g ["rm", "--quiet", "--", file]
|
|
-- git rm deletes empty directories; put them back
|
|
liftIO $ createDirectoryIfMissing True (parentDir file)
|
|
|
|
fromAnnex key file
|
|
logStatus key ValueMissing
|
|
|
|
return True
|