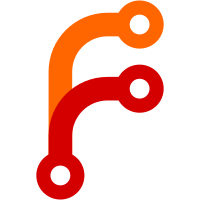
Improve disk free space checking when transferring unsized keys to local git remotes. Since the size of the object file is known, can check that instead. Getting unsized keys from local git remotes does not check the actual object size. It would be harder to handle that direction because the size check is run locally, before anything involving the remote is done. So it doesn't know the size of the file on the remote. Also, transferring unsized keys to other remotes, including ssh remotes and p2p remotes don't do disk size checking for unsized keys. This would need a change in protocol. (It does seem like it would be possible to implement the same thing for directory special remotes though.) In some sense, it might be better to not ever do disk free checking for unsized keys, than to do it only sometimes. A user might notice this direction working and consider it a bug that the other direction does not. On the other hand, disk reserve checking is not implemented for most special remotes at all, and yet it is implemented for a few, which is also inconsistent, but best effort. And so doing this best effort seems to make some sense. Fundamentally, if the user wants the size to always be checked, they should not use unsized keys. Sponsored-by: Brock Spratlen on Patreon
52 lines
1.4 KiB
Haskell
52 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010, 2015 Joey Hess <joey@kitenet.net>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.SetKey where
|
|
|
|
import Command
|
|
import Logs.Location
|
|
import Annex.Content
|
|
|
|
cmd :: Command
|
|
cmd = command "setkey" SectionPlumbing "sets annexed content for a key"
|
|
(paramPair paramKey paramPath)
|
|
(withParams seek)
|
|
|
|
seek :: CmdParams -> CommandSeek
|
|
seek = withWords (commandAction . start)
|
|
|
|
start :: [String] -> CommandStart
|
|
start ps@(keyname:file:[]) = starting "setkey" ai si $
|
|
perform file' (keyOpt keyname)
|
|
where
|
|
ai = ActionItemOther (Just (QuotedPath file'))
|
|
si = SeekInput ps
|
|
file' = toRawFilePath file
|
|
start _ = giveup "specify a key and a content file"
|
|
|
|
keyOpt :: String -> Key
|
|
keyOpt = fromMaybe (giveup "bad key") . deserializeKey
|
|
|
|
perform :: RawFilePath -> Key -> CommandPerform
|
|
perform file key = do
|
|
-- the file might be on a different filesystem, so moveFile is used
|
|
-- rather than simply calling moveAnnex; disk space is also
|
|
-- checked this way.
|
|
ok <- getViaTmp RetrievalAllKeysSecure DefaultVerify key (AssociatedFile Nothing) Nothing $ \dest -> unVerified $
|
|
if dest /= file
|
|
then liftIO $ catchBoolIO $ do
|
|
moveFile file dest
|
|
return True
|
|
else return True
|
|
if ok
|
|
then next $ cleanup key
|
|
else giveup "move failed!"
|
|
|
|
cleanup :: Key -> CommandCleanup
|
|
cleanup key = do
|
|
logStatus key InfoPresent
|
|
return True
|