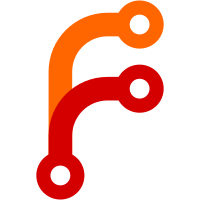
However, filepath-bytestring is still in Setup-Depends. That's because Utility.OsPath uses it when not built with OsPath. It would be maybe possible to make Utility.OsPath fall back to using filepath, and eliminate that dependency too, but it would mean either wrapping all of System.FilePath's functions, or using `type OsPath = FilePath` Annex.Import uses ifdefs to avoid converting back to FilePath when not on windows. On windows it's a bit slower due to that conversion. Utility.Path.Windows.convertToWindowsNativeNamespace got a bit slower too, but not really worth optimising I think. Note that importing Utility.FileSystemEncoding at the same time as System.Posix.ByteString will result in conflicting definitions for RawFilePath. filepath-bytestring avoids that by importing RawFilePath from System.Posix.ByteString, but that's not possible in Utility.FileSystemEncoding, since Setup-Depends does not include unix. This turned out not to affect any code in git-annex though. Sponsored-by: Leon Schuermann
46 lines
1.1 KiB
Haskell
46 lines
1.1 KiB
Haskell
{- Portability shim for touching a file.
|
|
-
|
|
- Copyright 2011-2018 Joey Hess <id@joeyh.name>
|
|
-
|
|
- License: BSD-2-clause
|
|
-}
|
|
|
|
{-# LANGUAGE CPP #-}
|
|
|
|
module Utility.Touch (
|
|
touchBoth,
|
|
touch
|
|
) where
|
|
|
|
#if ! defined(mingw32_HOST_OS)
|
|
|
|
import System.Posix.Files.ByteString
|
|
import Data.Time.Clock.POSIX
|
|
|
|
import Utility.RawFilePath
|
|
|
|
{- Changes the access and modification times of an existing file.
|
|
Can follow symlinks, or not. -}
|
|
touchBoth :: RawFilePath -> POSIXTime -> POSIXTime -> Bool -> IO ()
|
|
touchBoth file atime mtime follow
|
|
| follow = setFileTimesHiRes file atime mtime
|
|
| otherwise = setSymbolicLinkTimesHiRes file atime mtime
|
|
|
|
{- Changes the access and modification times of an existing file
|
|
- to the same value. Can follow symlinks, or not. -}
|
|
touch :: RawFilePath -> POSIXTime -> Bool -> IO ()
|
|
touch file mtime = touchBoth file mtime mtime
|
|
|
|
#else
|
|
|
|
import Data.Time.Clock.POSIX
|
|
import Utility.RawFilePath
|
|
|
|
{- Noop for Windows -}
|
|
touchBoth :: RawFilePath -> POSIXTime -> POSIXTime -> Bool -> IO ()
|
|
touchBoth _ _ _ _ = return ()
|
|
|
|
touch :: RawFilePath -> POSIXTime -> Bool -> IO ()
|
|
touch _ _ _ = return ()
|
|
|
|
#endif
|