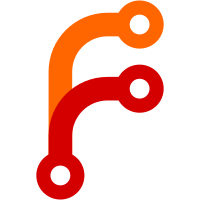
This avoids starting one process when only the other one is needed. Eg in git-annex smudge --clean, this reduces the total number of cat-file processes that are started from 4 to 2. The only performance penalty is that when both are needed, it has to do twice as much work to maintain the two Maps. But both are very small, consisting of 1 or 2 items, so that work is negligible. Sponsored-by: Dartmouth College's Datalad project
38 lines
972 B
Haskell
38 lines
972 B
Haskell
{- git-cat file handles pools
|
|
-
|
|
- Copyright 2020-2021 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Types.CatFileHandles (
|
|
CatFileHandles(..),
|
|
catFileHandlesNonConcurrent,
|
|
catFileHandlesPool,
|
|
CatMap(..),
|
|
emptyCatMap,
|
|
) where
|
|
|
|
import Control.Concurrent.STM
|
|
import qualified Data.Map as M
|
|
|
|
import Utility.ResourcePool
|
|
import Git.CatFile (CatFileHandle, CatFileMetaDataHandle)
|
|
|
|
data CatFileHandles
|
|
= CatFileHandlesNonConcurrent CatMap
|
|
| CatFileHandlesPool (TMVar CatMap)
|
|
|
|
data CatMap = CatMap
|
|
{ catFileMap :: M.Map FilePath (ResourcePool CatFileHandle)
|
|
, catFileMetaDataMap :: M.Map FilePath (ResourcePool CatFileMetaDataHandle)
|
|
}
|
|
|
|
emptyCatMap :: CatMap
|
|
emptyCatMap = CatMap M.empty M.empty
|
|
|
|
catFileHandlesNonConcurrent :: CatFileHandles
|
|
catFileHandlesNonConcurrent = CatFileHandlesNonConcurrent emptyCatMap
|
|
|
|
catFileHandlesPool :: IO CatFileHandles
|
|
catFileHandlesPool = CatFileHandlesPool <$> newTMVarIO emptyCatMap
|