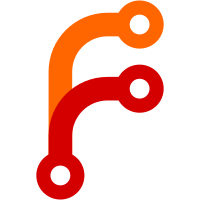
Added -z option to git-annex commands that use --batch, useful for supporting filenames containing newlines. It only controls input to --batch, the output will still be line delimited unless --json or etc is used to get some other output. While git often makes -z affect both input and output, I don't like trying them together, and making it affect output would have been a significant complication, and also git-annex output is generally not intended to be machine parsed, unless using --json or a format option. Commands that take pairs like "file key" still separate them with a space in --batch mode. All such commands take care to support filenames with spaces when parsing that, so there was no need to change it, and it would have needed significant changes to the batch machinery to separate tose with a null. To make fromkey and registerurl support -z, I had to give them a --batch option. The implicit batch mode they enter when not provided with input parameters does not support -z as that would have complicated option parsing. Seemed better to move these toward using the same --batch as everything else, though the implicit batch mode can still be used. This commit was sponsored by Ole-Morten Duesund on Patreon.
59 lines
1.4 KiB
Haskell
59 lines
1.4 KiB
Haskell
{- git-annex command
|
|
-
|
|
- Copyright 2010,2016 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU GPL version 3 or higher.
|
|
-}
|
|
|
|
module Command.DropKey where
|
|
|
|
import Command
|
|
import qualified Annex
|
|
import Logs.Location
|
|
import Annex.Content
|
|
|
|
cmd :: Command
|
|
cmd = noCommit $ withGlobalOptions [jsonOptions] $
|
|
command "dropkey" SectionPlumbing
|
|
"drops annexed content for specified keys"
|
|
(paramRepeating paramKey)
|
|
(seek <$$> optParser)
|
|
|
|
data DropKeyOptions = DropKeyOptions
|
|
{ toDrop :: [String]
|
|
, batchOption :: BatchMode
|
|
}
|
|
|
|
optParser :: CmdParamsDesc -> Parser DropKeyOptions
|
|
optParser desc = DropKeyOptions
|
|
<$> cmdParams desc
|
|
<*> parseBatchOption
|
|
|
|
seek :: DropKeyOptions -> CommandSeek
|
|
seek o = do
|
|
unlessM (Annex.getState Annex.force) $
|
|
giveup "dropkey can cause data loss; use --force if you're sure you want to do this"
|
|
withKeys start (toDrop o)
|
|
case batchOption o of
|
|
Batch fmt -> batchInput fmt parsekey $ batchCommandAction . start
|
|
NoBatch -> noop
|
|
where
|
|
parsekey = maybe (Left "bad key") Right . file2key
|
|
|
|
start :: Key -> CommandStart
|
|
start key = do
|
|
showStartKey "dropkey" key (mkActionItem key)
|
|
next $ perform key
|
|
|
|
perform :: Key -> CommandPerform
|
|
perform key = ifM (inAnnex key)
|
|
( lockContentForRemoval key $ \contentlock -> do
|
|
removeAnnex contentlock
|
|
next $ cleanup key
|
|
, next $ return True
|
|
)
|
|
|
|
cleanup :: Key -> CommandCleanup
|
|
cleanup key = do
|
|
logStatus key InfoMissing
|
|
return True
|