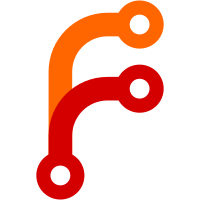
Mostly the username is only used for the git committer or other display purposes, and we can just fall back to a dummy value in these cases. The only remaining place where an error is thrown is when starting local pairing, which needs the username to be known.
34 lines
1.1 KiB
Haskell
34 lines
1.1 KiB
Haskell
{- git-annex assistant gpg stuff
|
|
-
|
|
- Copyright 2013 Joey Hess <id@joeyh.name>
|
|
-
|
|
- Licensed under the GNU AGPL version 3 or higher.
|
|
-}
|
|
|
|
module Assistant.Gpg where
|
|
|
|
import Utility.Gpg
|
|
import Utility.UserInfo
|
|
import Types.Remote (RemoteConfigKey)
|
|
|
|
import qualified Data.Map as M
|
|
|
|
{- Generates a gpg user id that is not used by any existing secret key -}
|
|
newUserId :: GpgCmd -> IO UserId
|
|
newUserId cmd = do
|
|
oldkeys <- secretKeys cmd
|
|
username <- either (const "unknown") id <$> myUserName
|
|
let basekeyname = username ++ "'s git-annex encryption key"
|
|
return $ Prelude.head $ filter (\n -> M.null $ M.filter (== n) oldkeys)
|
|
( basekeyname
|
|
: map (\n -> basekeyname ++ show n) ([2..] :: [Int])
|
|
)
|
|
|
|
data EnableEncryption = HybridEncryption | SharedEncryption | NoEncryption
|
|
deriving (Eq)
|
|
|
|
{- Generates Remote configuration for encryption. -}
|
|
configureEncryption :: EnableEncryption -> (RemoteConfigKey, String)
|
|
configureEncryption SharedEncryption = ("encryption", "shared")
|
|
configureEncryption NoEncryption = ("encryption", "none")
|
|
configureEncryption HybridEncryption = ("encryption", "hybrid")
|